Python: Get a list with n elements removed from the left, right
Remove N Elements from Sides
Write a Python program to get a list with n elements removed from the left and right.
Removed from the left:
- Use slice notation to remove the specified number of elements from the left.
- Omit the last argument, n, to use a default value of 1.
Removed from the right:
Returns a list with n elements removed from the right.
- Use slice notation to remove the specified number of elements from the right.
- Omit the last argument, n, to use a default value of 1.
Sample Solution:
Python Code:
# Define a function named 'drop_left_right' that removes elements from the left and right of a list.
# It takes a list 'a' and an optional parameter 'n' specifying the number of elements to remove from both sides.
def drop_left_right(a, n=1):
# Return a tuple of two lists: elements from index 'n' to the end and elements from the beginning to 'n' from the original list 'a'.
return a[n:], a[:-n]
# Create a sample list 'nums' with integer elements.
nums = [1, 2, 3]
# Print the original list elements.
print("Original list elements:")
print(nums)
# Use the 'drop_left_right' function to remove 1 element from the left of the list and store the results in 'result'.
result = drop_left_right(nums)
# Print the list with 1 element removed from the left.
print("Remove 1 element from the left of the said list:")
print(result[0])
# Print the list with 1 element removed from the right.
print("Remove 1 element from the right of the said list:")
print(result[1])
# Create another sample list 'nums' with integer elements.
nums = [1, 2, 3, 4]
# Print the original list elements.
print("\nOriginal list elements:")
print(nums)
# Use the 'drop_left_right' function to remove 2 elements from the left of the list and store the results in 'result'.
result = drop_left_right(nums, 2)
# Print the list with 2 elements removed from the left.
print("Remove 2 elements from the left of the said list:")
print(result[0])
# Print the list with 2 elements removed from the right.
print("Remove 2 elements from the right of the said list:")
print(result[1])
# Create another sample list 'nums' with integer elements.
nums = [1, 2, 3, 4, 5, 6]
# Print the original list elements.
print("\nOriginal list elements:")
print(nums)
# Use the 'drop_left_right' function without specifying 'n' to remove 1 element from both left and right.
result = drop_left_right(nums)
# Print the list with 1 element removed from both left and right.
print("Remove 1 element from both left and right of the said list:")
print(result[0])
print("Remove 1 element from both left and right of the said list:")
print(result[1])
Sample Output:
Original list elements: [1, 2, 3] Remove 1 element from left of the said list: [2, 3] Remove 1 element from right of the said list: [1, 2] Original list elements: [1, 2, 3, 4] Remove 2 elements from left of the said list: [3, 4] Remove 2 elements from right of the said list: [1, 2] Original list elements: [1, 2, 3, 4, 5, 6] Remove 7 elements from left of the said list: [2, 3, 4, 5, 6] Remove 7 elements from right of the said list: [1, 2, 3, 4, 5]
Flowchart:
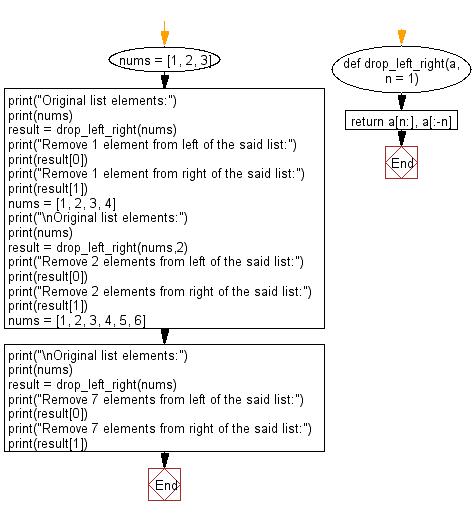
For more Practice: Solve these Related Problems:
- Write a Python program to remove n elements from both the left and right ends of a list.
- Write a Python program to remove a specified percentage of elements from both ends of a list.
- Write a Python program to remove n elements from the start and a different number m from the end of a list.
- Write a Python program to remove n elements from each side and return an empty list if n exceeds half the list's length.
Go to:
Previous: Write a Python program to get the cumulative sum of the elements of a given list.
Next: Write a Python program to get the every nth element in a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.