Python: Get every nth element in a given list
Python List: Exercise - 269 with Solution
Write a Python program to get every nth element in a given list.
Use slice notation to create a new list that contains every nth element of the given list
Sample Solution:
Python Code:
# Define a function named 'every_nth' that returns every nth element from a list.
# It takes two parameters: 'nums' (the list) and 'nth' (the interval for selecting elements).
def every_nth(nums, nth):
# Use list slicing to return elements starting from the (nth-1) index, with a step of 'nth'.
return nums[nth - 1::nth]
# Create a sample list of integers from 1 to 10.
nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Print the original list elements.
print(every_nth(nums, 1)) # Select every 1st element (no change).
print(every_nth(nums, 2)) # Select every 2nd element.
print(every_nth(nums, 5)) # Select every 5th element.
print(every_nth(nums, 6)) # Select every 6th element.
Sample Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10] [2, 4, 6, 8, 10] [5, 10] [6]
Flowchart:
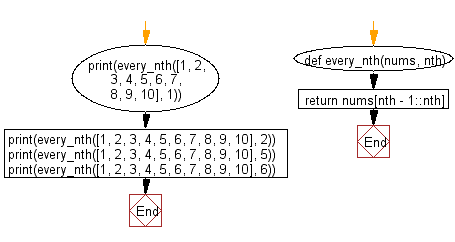
Python Code Editor:
Previous: Write a Python program to get a list with n elements removed from the left, right.
Next: Write a Python program to check if the elements of the first list are contained in the second one regardless of order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-269.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics