Python Exercises: Find the largest odd number in a list of integers
Find Largest Odd Number
Write a Python program to find the largest odd number in a given list of integers.
Sample Data:
([0, 9, 2, 4, 5, 6]) -> 9
([-4, 0, 6, 1, 0, 2]) -> 1
([1, 2, 3]) -> 3
([-4, 0, 5, 1, 0, 1]) -> 5
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes a list 'nums' as input.
def test(nums):
# Use a list comprehension to filter out odd numbers (numbers not divisible by 2) from the input list 'nums'.
odd_nums = [x for x in nums if x % 2 != 0]
# Check if the list 'odd_nums' is empty, meaning there are no odd numbers in the 'nums' list.
if len(odd_nums) == 0:
# If there are no odd numbers, return -1 as a sentinel value to indicate no odd numbers were found.
return -1
else:
# If there are odd numbers, find and return the maximum (largest) odd number in the 'odd_nums' list.
return max(odd_nums)
# Define the original list 'nums'.
nums = [0, 9, 2, 4, 5, 6]
print("Original list:")
print(nums)
# Call the 'test' function to find the largest odd number in the list.
print("Find the largest odd number in the said list:", test(nums))
# Define another list 'nums'.
nums = [-4, 0, 6, 1, 0, 2]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the second list of numbers.
print("Find the largest odd number in the said list:", test(nums))
# Define another list 'nums'.
nums = [1, 2, 3]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the third list of numbers.
print("Find the largest odd number in the said list:", test(nums))
# Define another list 'nums'.
nums = [-4, 0, 5, 1, 0, 1]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the fourth list of numbers.
print("Find the largest odd number in the said list:", test(nums))
Sample Output:
Original list: [0, 9, 2, 4, 5, 6] Find the largest odd number in the said list: 9 Original list: [-4, 0, 6, 1, 0, 2] Find the largest odd number in the said list: 1 Original list: [1, 2, 3] Find the largest odd number in the said list: 3 Original list: [-4, 0, 5, 1, 0, 1] Find the largest odd number in the said list: 5
Flowchart:
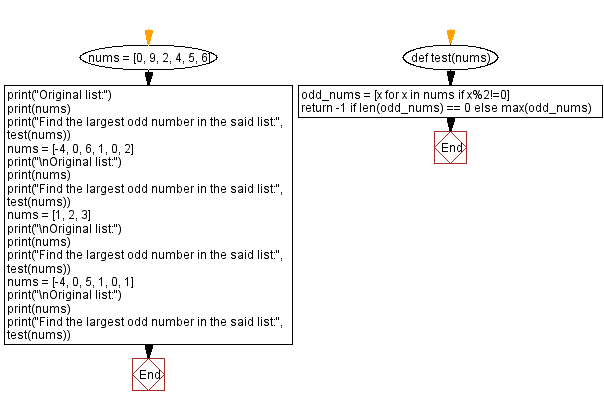
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes a list 'nums' as input.
def test(nums):
# Initialize the 'result' variable to -1, which serves as a sentinel value.
result = -1
# Iterate through the elements 'x' in the input list 'nums'.
for x in nums:
# Check if 'x' is an odd number (not divisible by 2) and greater than the current 'result'.
if x % 2 and x > result:
# If both conditions are met, update 'result' to the current 'x' value.
result = x
# Return the final value of 'result,' which is the largest odd number in the list.
return result
# Define the original list 'nums'.
nums = [0, 9, 2, 4, 5, 6]
print("Original list:")
print(nums)
# Call the 'test' function to find the largest odd number in the list.
print("Find the largest odd number in the said list:", test(nums))
# Define another list 'nums'.
nums = [-4, 0, 6, 1, 0, 2]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the second list of numbers.
print("Find the largest odd number in the said list:", test(nums))
# Define another list 'nums'.
nums = [1, 2, 3]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the third list of numbers.
print("Find the largest odd number in the said list:", test(nums))
# Define another list 'nums'.
nums = [-4, 0, 5, 1, 0, 1]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the fourth list of numbers.
print("Find the largest odd number in the said list:", test(nums))
Sample Output:
Original list: [0, 9, 2, 4, 5, 6] Find the largest odd number in the said list: 9 Original list: [-4, 0, 6, 1, 0, 2] Find the largest odd number in the said list: 1 Original list: [1, 2, 3] Find the largest odd number in the said list: 3 Original list: [-4, 0, 5, 1, 0, 1] Find the largest odd number in the said list: 5
Flowchart:
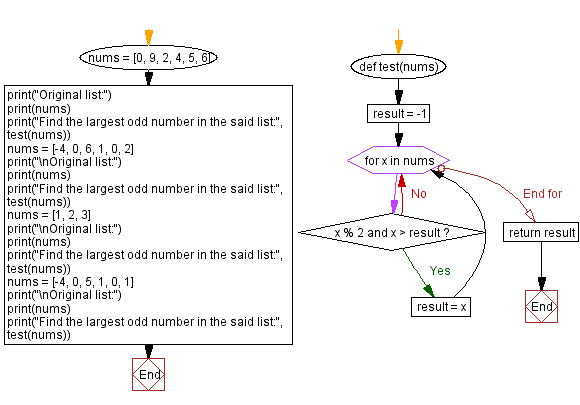
For more Practice: Solve these Related Problems:
- Write a Python program to find the smallest odd number in a list of integers.
- Write a Python program to retrieve the largest odd number from a list after excluding numbers divisible by a specified value.
- Write a Python program to return both the largest odd number and its index within the list.
- Write a Python program to find the largest odd number in a list and return a custom message if none exist.
Go to:
Previous Python Exercise: Sum of all list elements except current element.
Next Python Exercise: Largest, lowest gap between sorted values of a list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.