Python Exercises: Sum of missing numbers of a list of integers
Sum Missing Numbers
Write a Python program to sum the missing numbers in a given list of integers.
Sample Data:
([0, 3, 4, 7, 9]) -> 22
([44, 45, 48]) -> 93
([-7, -5, -4, 0]) -> -12
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes a list 'nums' as input.
def test(nums):
# Find the maximum and minimum numbers in the input list 'nums'.
max_num = max(nums)
min_num = min(nums)
# Calculate the sum of missing numbers within the range [min_num, max_num] using the formula:
# Sum = (max + min) * (max - min + 1) // 2 - sum(nums)
return (max_num + min_num) * (max_num - min_num + 1) // 2 - sum(nums)
# Define the original list 'nums'.
nums = [0, 3, 4, 7, 9]
print("Original list:")
print(nums)
# Call the 'test' function to calculate the sum of missing numbers in the list of integers.
print("Sum of missing numbers of the said list of integers:", test(nums))
# Define another list 'nums'.
nums = [44, 45, 48]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the second list of integers.
print("Sum of missing numbers of the said list of integers:", test(nums))
# Define another list 'nums'.
nums = [-7, -5, -4, 0]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the third list of integers.
print("Sum of missing numbers of the said list of integers:", test(nums))
Sample Output:
Original list: [0, 3, 4, 7, 9] Sum of missing numbers of the said list of integers: 22 Original list: [44, 45, 48] Sum of missing numbers of the said list of integers: 93 Original list: [-7, -5, -4, 0] Sum of missing numbers of the said list of integers: -12
Flowchart:
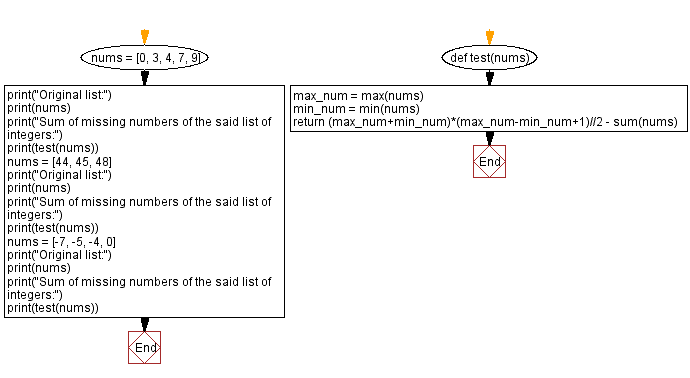
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes a list 'nums' as input.
def test(nums):
# Find the maximum and minimum numbers in the input list 'nums'.
max_num = max(nums)
min_num = min(nums)
# Calculate the sum of missing numbers within the range [min_num, max_num] using the formula:
# Sum = sum(range(min_num, max_num + 1)) - sum(nums)
return sum(range(min_num, max_num + 1)) - sum(nums)
# Define the original list 'nums'.
nums = [0, 3, 4, 7, 9]
print("Original list:")
print(nums)
# Call the 'test' function to calculate the sum of missing numbers in the list of integers.
print("Sum of missing numbers of the said list of integers:", test(nums))
# Define another list 'nums'.
nums = [44, 45, 48]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the second list of integers.
print("Sum of missing numbers of the said list of integers:", test(nums))
# Define another list 'nums'.
nums = [-7, -5, -4, 0]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the third list of integers.
print("Sum of missing numbers of the said list of integers:", test(nums))
Sample Output:
Original list: [0, 3, 4, 7, 9] Sum of missing numbers of the said list of integers: 22 Original list: [44, 45, 48] Sum of missing numbers of the said list of integers: 93 Original list: [-7, -5, -4, 0] Sum of missing numbers of the said list of integers: -12
Flowchart:
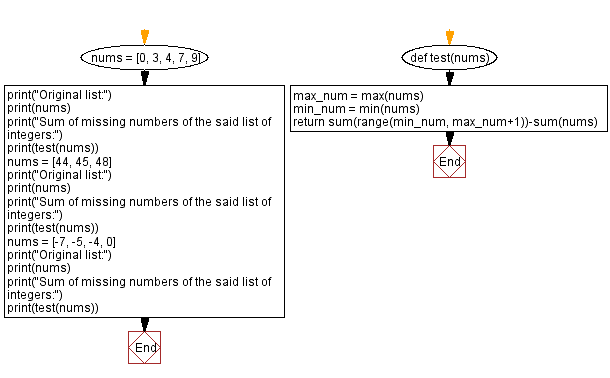
For more Practice: Solve these Related Problems:
- Write a Python program to sum all missing numbers between the minimum and maximum values in a list.
- Write a Python program to compute the sum of missing even numbers in a given list.
- Write a Python program to identify missing numbers in a sorted list and return both the missing numbers and their total sum.
- Write a Python program to calculate the sum of missing numbers in a list after removing duplicate entries.
Go to:
Previous Python Exercise: Largest, lowest gap between sorted values of a list.
Next Python Exercise: Check if each number is prime in a list of numbers.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.