Python: Create a list by concatenating a given list which range goes from 1 to n
Create List with Range Concatenation
Write a Python program to create a list by concatenating a given list with a range from 1 to n.
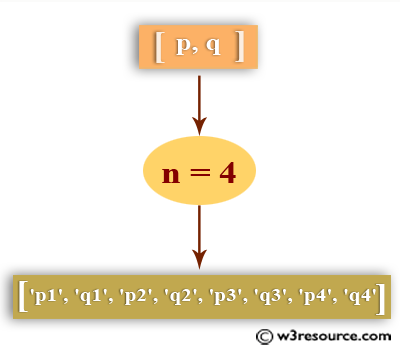
Sample Solution:
Python Code:
# Define a list 'my_list' containing elements 'p' and 'q'
my_list = ['p', 'q']
# Define a variable 'n' with the value 4
n = 4
# Use a list comprehension to create a new list 'new_list' by combining elements from 'my_list' with numbers from 1 to 'n'
new_list = ['{}{}'.format(x, y) for y in range(1, n+1) for x in my_list]
# Print the 'new_list' containing combinations of elements from 'my_list' and numbers
print(new_list)
Sample Output:
['p1', 'q1', 'p2', 'q2', 'p3', 'q3', 'p4', 'q4']
Flowchart:
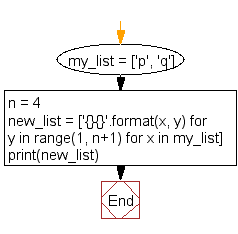
For more Practice: Solve these Related Problems:
- Write a Python program to create a list by concatenating two lists element-wise.
- Write a Python program to generate a list by concatenating words from a list with numbers from a range.
- Write a Python program to create a list of strings by appending a given suffix to each element.
- Write a Python program to create a list with all possible concatenations of two given lists.
Go to:
Previous: Write a Python program using Sieve of Eratosthenes method for computing primes upto a specified number.
Next: Write a Python program to get variable unique identification number or string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.