Python: Find missing and additional values in two lists
Python List: Exercise - 42 with Solution
Write a Python program to find missing and additional values in two lists.
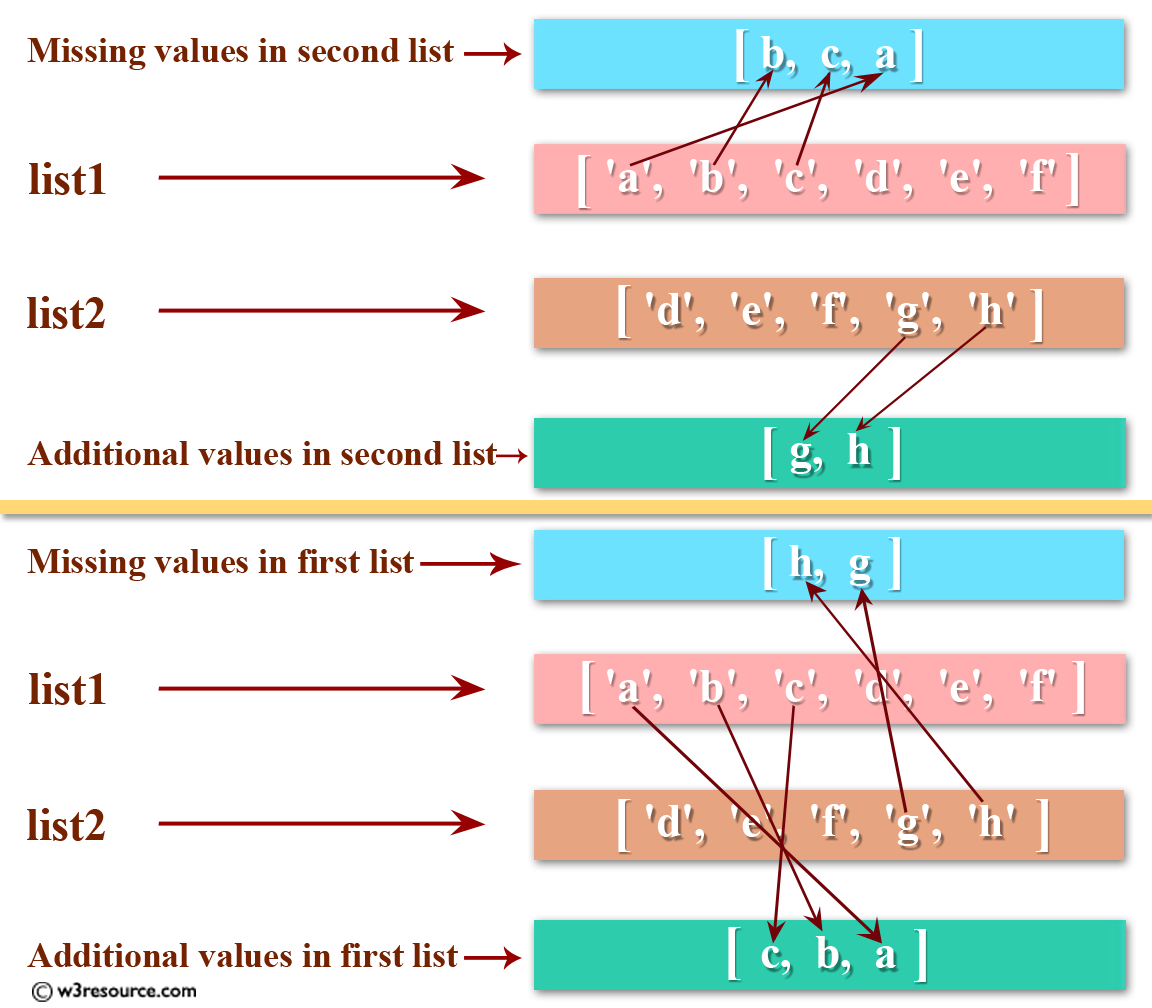
Sample Solution:
Python Code :
# Define two lists 'list1' and 'list2' containing elements
list1 = ['a', 'b', 'c', 'd', 'e', 'f']
list2 = ['d', 'e', 'f', 'g', 'h']
# Calculate the missing values in 'list2' by finding the set difference between 'list1' and 'list2'
missing_values = set(list1).difference(list2)
# Print the missing values in the second list 'list2'
print('Missing values in the second list: ', ','.join(missing_values))
# Calculate the additional values in 'list2' by finding the set difference between 'list2' and 'list1'
additional_values = set(list2).difference(list1)
# Print the additional values in the second list 'list2'
print('Additional values in the second list: ', ','.join(additional_values))
Sample Output:
Missing values in second list: b,c,a Additional values in second list: g,h
Flowchart:
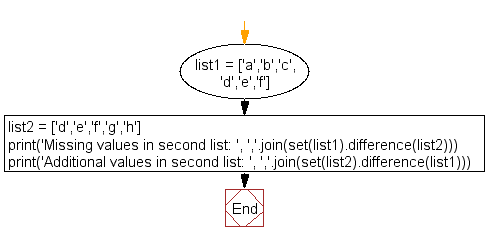
Python Code Editor:
Previous: Write a Python program to create multiple lists.
Next: Write a Python program to split a list into different variables.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-42.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics