Python: Compute the difference between two lists
Difference Between Two Lists
Write a Python program to compute the difference between two lists.
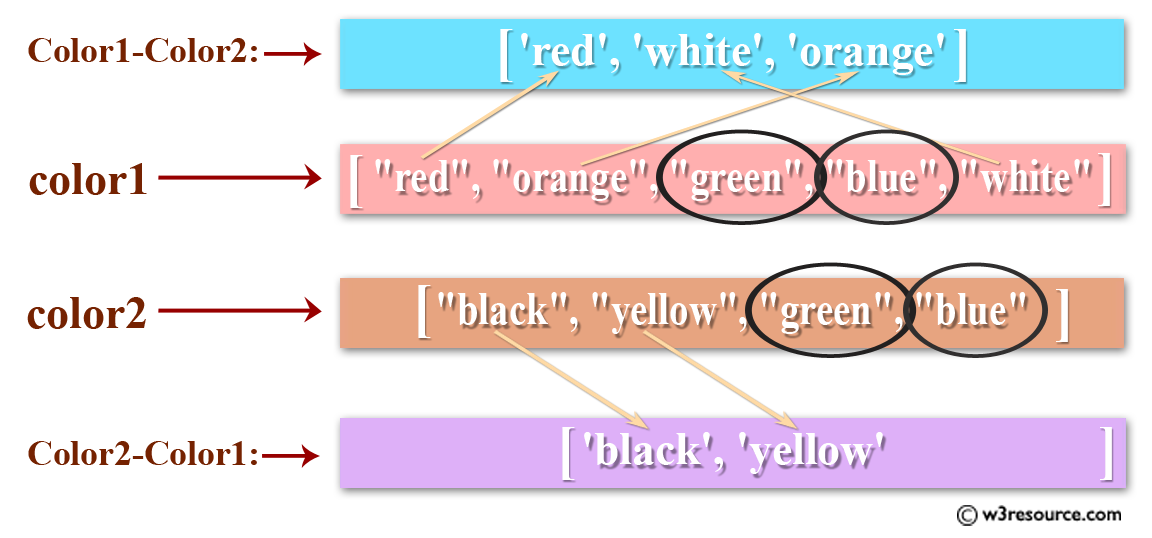
Sample Solution:
Python Code :
# Import the 'Counter' class from the 'collections' module
from collections import Counter
# Define two lists 'color1' and 'color2' containing color names
color1 = ["red", "orange", "green", "blue", "white"]
color2 = ["black", "yellow", "green", "blue"]
# Create Counter objects 'counter1' and 'counter2' for each list to count the occurrences of color names
counter1 = Counter(color1)
counter2 = Counter(color2)
# Print the elements that are in 'counter1' but not in 'counter2' (Color1-Color2)
print("Color1-Color2: ", list(counter1 - counter2))
# Print the elements that are in 'counter2' but not in 'counter1' (Color2-Color1)
print("Color2-Color1: ", list(counter2 - counter1))
Sample Output:
Color1-Color2: ['red', 'white', 'orange'] Color2-Color1: ['black', 'yellow']
Flowchart:
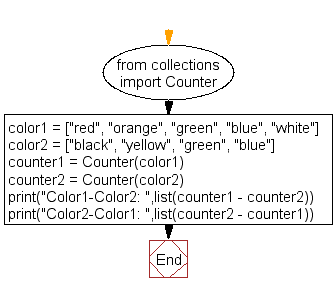
Python Code Editor:
Previous: Write a Python program to split a list every Nth element.
Next: Write a Python program to create a list with infinite elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics