Python: Convert a string to a list
Convert String to List
Write a Python program to convert a string to a list.
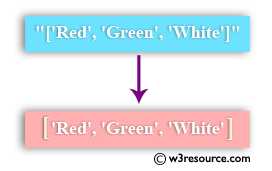
Sample Solution:
Python Code:
# Import the 'ast' module, which provides a safe way to evaluate Python literals
import ast
# Define a string 'color' containing a Python list as a string
color = "['Red', 'Green', 'White']"
# Use the 'ast.literal_eval' function to safely evaluate the string as a Python literal expression
# This function ensures that the string is treated as a valid Python literal, in this case, a list
# Print the result, which is the actual list created from the string
print(ast.literal_eval(color))
Sample Output:
['Red', 'Green', 'White']
Flowchart:
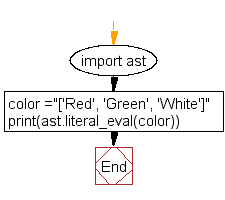
Python Code Editor:
Previous: Write a Python program to remove key values pairs from a list of dictionaries.
Next: Write a Python program to check if all items of a given list of strings is equal to a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics