Python: Check whether all items of a list is equal to a given string
Check All Strings Match Given String
Write a Python program to check if all items in a given list of strings are equal to a given string.
Sample Solution-1:
Python Code:
# Define a list 'color1' containing color names
color1 = ["green", "orange", "black", "white"]
# Define another list 'color2' containing color names, with multiple occurrences of 'green'
color2 = ["green", "green", "green", "green"]
# Check if all elements in 'color1' are equal to 'blue' using a generator expression and the 'all' function
# The 'all' function returns True if all elements in the iterable are True (in this case, if all elements are 'blue')
# Since there is no 'blue' in 'color1', this will return False
print(all(c == 'blue' for c in color1))
# Check if all elements in 'color2' are equal to 'green' using a generator expression and the 'all' function
# The 'all' function returns True if all elements in the iterable are True (in this case, if all elements are 'green')
# Since all elements in 'color2' are 'green', this will return True
print(all(c == 'green' for c in color2))
Sample Output:
False True
Sample Solution-2:
Checks if all elements in a list are equal:
Use set() to eliminate duplicate elements and then use len() to check if length is 1.
Python Code:
# Define a function 'all_equal' that takes a list 'nums' as an argument
def all_equal(nums):
# Convert the list 'nums' to a set to remove duplicates, then calculate its length
# If the length is 1, it means all elements in the list are equal
return len(set(nums)) == 1
# Call the 'all_equal' function with a list of numbers and print the result
print(all_equal([1, 2, 3, 4, 5, 6]))
# Call the 'all_equal' function with a list of identical numbers and print the result
print(all_equal([4, 4, 4, 4, 4]))
Sample Output:
False True
Flowchart:
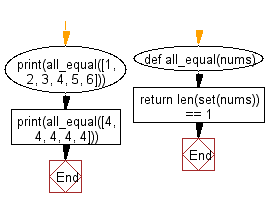
For more Practice: Solve these Related Problems:
- Write a Python program to check if a given list of strings contains at least one string that matches a given value.
- Write a Python program to find all elements in a list of strings that contain a given substring.
- Write a Python program to check if a list of strings contains at least one string starting with a given prefix.
- Write a Python program to count how many times a given string appears in a list of strings.
Go to:
Previous: Write a Python program to convert a string to a list.
Next: Write a Python program to replace the last element in a list with another list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.