Python: Find a tuple, the smallest second index value from a list of tuples
Smallest Second Index Tuple
Write a Python program to find a tuple, the smallest second index value from a list of tuples.
Visual Presentation:
Sample Solution:
Python Code:
# Define a list 'x' containing tuples, where each tuple has two elements
x = [(4, 1), (1, 2), (6, 0)]
# Use the 'min' function to find the minimum element in the list 'x' based on a custom key
# The 'key' argument specifies a lambda function that calculates a key for each element in 'x'
# The lambda function computes the key as a tuple, where the first element is the second element of the tuple (n[1]),
# and the second element is the negation of the first element (-n[0])
# This effectively sorts the tuples first by their second element in ascending order and then by their first element in descending order
# The 'min' function returns the tuple with the minimum key value
print(min(x, key=lambda n: (n[1], -n[0])))
Sample Output:
(6, 0)
Flowchart:
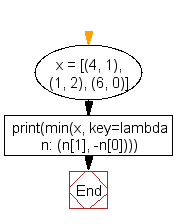
For more Practice: Solve these Related Problems:
- Write a Python program to find a tuple with the largest first index value from a list of tuples.
- Write a Python program to remove all tuples from a list where the second index value is smaller than a given number.
- Write a Python program to sort a list of tuples based on the sum of elements in each tuple.
- Write a Python program to find the tuple with the highest product of elements in a list of tuples.
Go to:
Previous: Write a Python program to check if the n-th element exists in a given list.
Next: Write a Python program to create a list of empty dictionaries.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.