Python: Iterate over two lists simultaneously
Python List: Exercise - 64 with Solution
Write a Python program to iterate over two lists simultaneously.
Visual Presentation:
Sample Solution-1:
Python Code:
# Define two lists 'num' and 'color' containing integers and color names, respectively
num = [1, 2, 3]
color = ['red', 'white', 'black']
# Use a 'for' loop and the 'zip' function to iterate over pairs of elements from 'num' and 'color' in parallel
# In each iteration, the variables 'a' and 'b' will hold the values from 'num' and 'color', respectively
# Print the values of 'a' and 'b' in each iteration
for (a, b) in zip(num, color):
print(a, b)
Sample Output:
1 red 2 white 3 black
Flowchart:
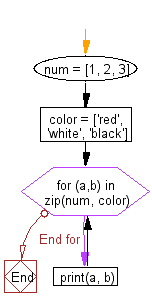
Sample Solution-2:
Python Code:
# Define a function 'print_itr' that takes three arguments: 'itr1', 'itr2', and 'fn'
def print_itr(itr1, itr2, fn):
# Use a 'for' loop and the 'zip' function to iterate over pairs of elements from 'itr1' and 'itr2' in parallel
for (itr1, itr2) in zip(num, color):
# Call the function 'fn' with 'itr1' and 'itr2' as arguments
fn(itr1, itr2)
# Define two lists 'num' and 'color' containing integers and color names, respectively
num = [1, 2, 3]
color = ['red', 'white', 'black']
# Call the 'print_itr' function with 'num', 'color', and the 'print' function as arguments
# This will print the pairs of elements from 'num' and 'color using the 'print' function
print_itr(num, color, print)
Sample Output:
1 red 2 white 3 black
Flowchart:
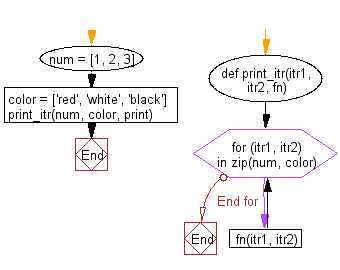
Python Code Editor:
Previous: Write a Python program to insert a given string at the beginning of all items in a list.
Next: Write a Python program to move all zero digits to end of a given list of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/list/python-data-type-list-exercise-64.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics