Python: Insert an element at a specified position into a given list
Insert Element at Specified Position
Write a Python program to insert an element at a specified position into a given list.
Sample Solution:
Python Code:
# Define a function 'insert_spec_position' that takes an element 'x', a list 'n_list', and an integer 'pos' as input
def insert_spec_position(x, n_list, pos):
# Return a modified list by inserting the element 'x' at the specified position (pos-1) in the input list
return n_list[:pos - 1] + [x] + n_list[pos - 1:]
# Create a list 'n_list' containing integers
n_list = [1, 1, 2, 3, 4, 4, 5, 1]
# Print a message indicating the original list
print("Original list:")
# Print the original list
print(n_list)
# Assign an integer 'kth_position' with the value 3
kth_position = 3
# Assign an integer 'x' with the value 12
x = 12
# Call the 'insert_spec_position' function with 'x', 'n_list', and 'kth_position'
# and store the result in the 'result' variable
result = insert_spec_position(x, n_list, kth_position)
# Print a message indicating the list after inserting an element at the kth position
print("\nAfter inserting an element at kth position in the said list:")
# Print the 'result' list
print(result)
Sample Output:
Original list: [1, 1, 2, 3, 4, 4, 5, 1] After inserting an element at kth position in the said list: [1, 1, 12, 2, 3, 4, 4, 5, 1]
Flowchart:
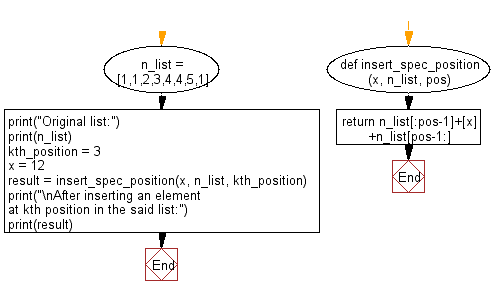
For more Practice: Solve these Related Problems:
- Write a Python program to insert multiple elements at specified positions in a list.
- Write a Python program to insert an element at a negative index position in a list.
- Write a Python program to insert an element at every K-th position in a list.
- Write a Python program to insert an element before every occurrence of a specified value in a list.
Go to:
Previous: Write a Python program to remove the K'th element from a given list, print the new list.
Next: Write a Python program to extract a given number of randomly selected elements from a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.