Python: Count number of lists in a given list of lists
Count Lists in Nested List
Write a Python program to count the number of lists in a given list of lists.
Visual Presentation:
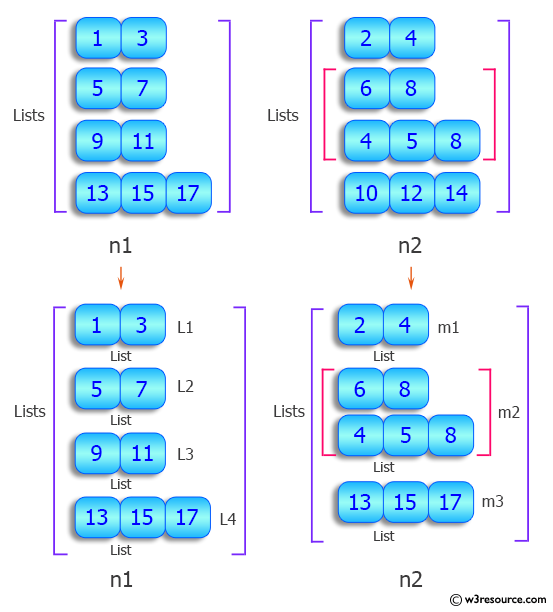
Sample Solution:
Python Code:
# Define a function 'count_list' that calculates the number of lists in the input list
def count_list(input_list):
return len(input_list)
# Create two lists 'list1' and 'list2' containing sublists of varying depths
list1 = [[1, 3], [5, 7], [9, 11], [13, 15, 17]]
list2 = [[2, 4], [[6, 8], [4, 5, 8]], [10, 12, 14]]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'list1'
print(list1)
# Print a message indicating the number of lists in the first list of lists
print("\nNumber of lists in said list of lists:")
# Call the 'count_list' function with 'list1' and print the result
print(count_list(list1))
# Print a message indicating the original list
print("\nOriginal list:")
# Print the contents of 'list2'
print(list2)
# Print a message indicating the number of lists in the second list of lists
print("\nNumber of lists in said list of lists:")
# Call the 'count_list' function with 'list2' and print the result
print(count_list(list2))
Sample Output:
Original list: [[1, 3], [5, 7], [9, 11], [13, 15, 17]] Number of lists in said list of lists: 4 Original list: [[2, 4], [[6, 8], [4, 5, 8]], [10, 12, 14]] Number of lists in said list of lists: 3
Flowchart:
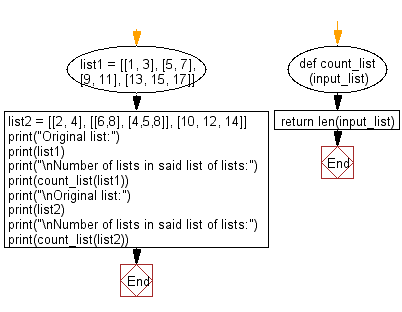
For more Practice: Solve these Related Problems:
- Write a Python program to count nested sublists within a list.
- Write a Python program to find the depth of a nested list.
- Write a Python program to check if all elements in a nested list are lists.
- Write a Python program to flatten a nested list and count unique elements.
Go to:
Previous: Write a Python program to Zip two given lists of lists.
Next: Write a Python program to find the list with maximum and minimum length.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.