Python: Sort a list of lists by length and value
Sort List of Lists by Length and Value
Write a Python program to sort a given list of lists by length and value.
Visual Presentation:
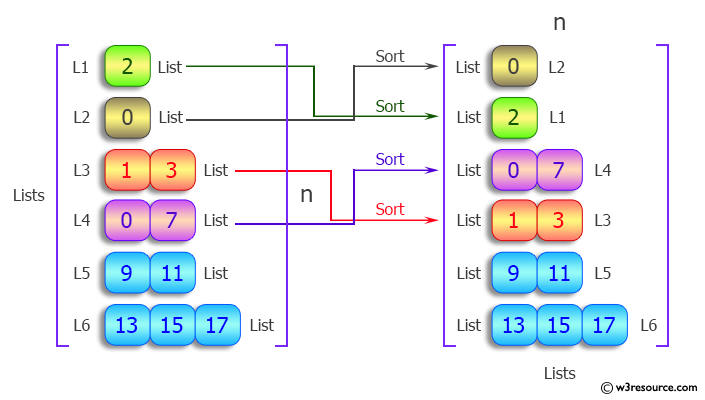
Sample Solution:
Python Code:
# Define a function 'sort_sublists' that sorts a list of lists by length and values
def sort_sublists(input_list):
input_list.sort() # Sort the list by sublist contents
input_list.sort(key=len) # Sort the list by the length of sublists
return input_list
# Create a list 'list1' containing sublists of numbers
list1 = [[2], [0], [1, 3], [0, 7], [9, 11], [13, 15, 17]]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'list1'
print(list1)
# Print a message indicating the sorted list of lists by length and values
print("\nSort the list of lists by length and value:")
# Call the 'sort_sublists' function with 'list1' and print the result
print(sort_sublists(list1))
Sample Output:
Original list: [[2], [0], [1, 3], [0, 7], [9, 11], [13, 15, 17]] Sort the list of lists by length and value: [[0], [2], [0, 7], [1, 3], [9, 11], [13, 15, 17]]
Flowchart:
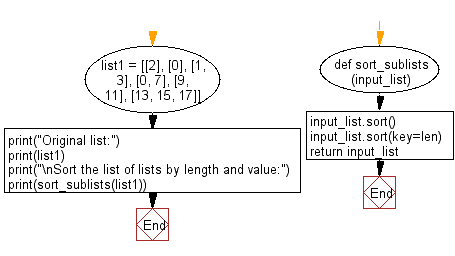
For more Practice: Solve these Related Problems:
- Write a Python program to sort a list of lists by the sum of its elements.
- Write a Python program to sort lists of lists by their maximum value.
- Write a Python program to reverse sort a list of lists by length.
- Write a Python program to order nested lists by ascending and descending order.
Go to:
Previous: Write a Python program to sort each sublist of strings in a given list of lists.
Next: Write a Python program to remove sublists from a given list of lists, which are outside a given range.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.