Python Math: Sum all amicable numbers from 1 to specified numbers
14. Amicable Numbers Sum
Write a Python program to sum all amicable numbers from 1 to specified numbers.
Note: Amicable numbers are two different numbers so related that the sum of the proper divisors of each is equal to the other number. (A proper divisor of a number is a positive factor of that number other than the number itself. For example, the proper divisors of 6 are 1, 2, and 3.)
Sample Solution :
Python Code :
def amicable_numbers_sum(limit):
if not isinstance(limit, int):
return "Input is not an integer!"
if limit < 1:
return "Input must be bigger than 0!"
amicables = set()
for num in range(2, limit+1):
if num in amicables:
continue
sum_fact = sum([fact for fact in range(1, num) if num % fact == 0])
sum_fact2 = sum([fact for fact in range(1, sum_fact) if sum_fact % fact == 0])
if num == sum_fact2 and num != sum_fact:
amicables.add(num)
amicables.add(sum_fact2)
return sum(amicables)
print(amicable_numbers_sum(9999))
print(amicable_numbers_sum(999))
print(amicable_numbers_sum(99))
Sample Output:
31626 504 0
Pictorial Presentation:
Flowchart:
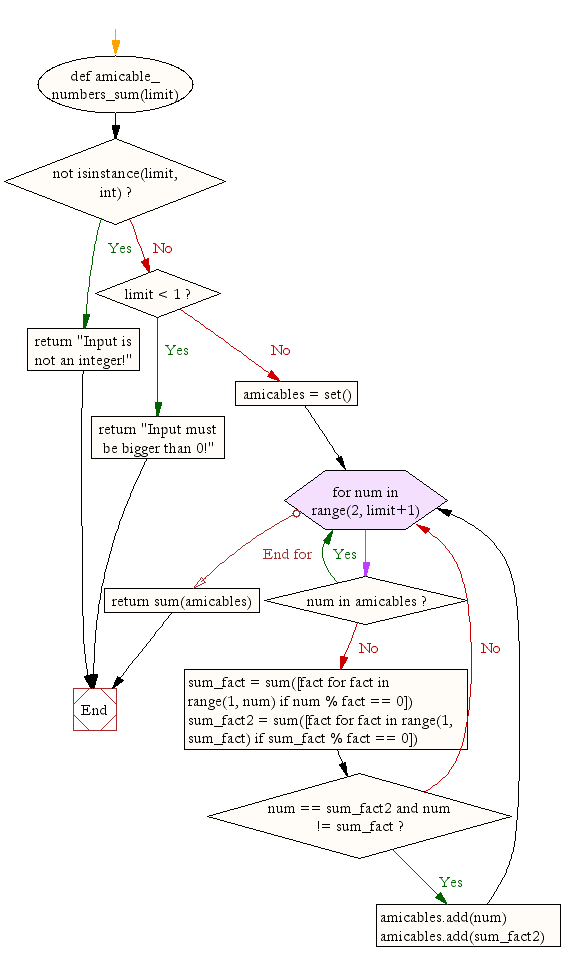
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the sum of proper divisors for a given number and then determine if it is amicable with any other number in a specified range.
- Write a Python function that accepts a number and returns the sum of its amicable pair if it exists, or 0 if not.
- Write a Python script to iterate through a list of numbers and print the amicable pair sums for each number that forms an amicable pair.
- Write a Python program to check if a number has an amicable pair by comparing its proper divisor sum to another number's divisor sum, and then print the amicable pair if found.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find out, if the given number is abundant.
Next: Write a Python program to returns sum of all divisors of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.