Python Math: Find the roots of a quadratic function
30. Quadratic Roots Finder
Write a Python program to find the roots of a quadratic function.
Sample Solution:
Python Code:
from math import sqrt
print("Quadratic function : (a * x^2) + b*x + c")
a = float(input("a: "))
b = float(input("b: "))
c = float(input("c: "))
r = b**2 - 4*a*c
if r > 0:
num_roots = 2
x1 = (((-b) + sqrt(r))/(2*a))
x2 = (((-b) - sqrt(r))/(2*a))
print("There are 2 roots: %f and %f" % (x1, x2))
elif r == 0:
num_roots = 1
x = (-b) / 2*a
print("There is one root: ", x)
else:
num_roots = 0
print("No roots, discriminant < 0.")
exit()
Sample Output:
Quadratic function : (a * x^2) + b*x + c a: 5 b: 20 c: 10 There are 2 roots: -0.585786 and -3.414214
Flowchart:
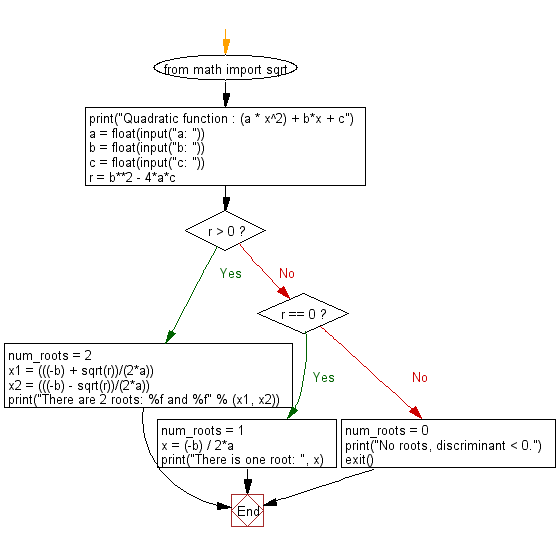
For more Practice: Solve these Related Problems:
- Write a Python program that calculates the roots of a quadratic equation using the quadratic formula, and prints both roots formatted to 6 decimal places.
- Write a Python function that takes coefficients a, b, and c, computes the roots (real or complex), and returns them as a tuple.
- Write a Python script to prompt the user for quadratic coefficients, then output the nature of the roots (real and distinct, real and equal, or complex) along with the computed values.
- Write a Python program to compare the roots of two quadratic equations and print which one has the larger positive root.
Go to:
Previous: Write a Python program to calculate wind chill index.
Next: Write a Python program to convert a binary number to decimal number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.