Python Math: Round a specified decimal by setting precision
40. Precision-Based Rounding of Decimal
Write a Python program to round a specified decimal by setting precision (between 1 and 4).
Sample Solution:
Python Code:
import decimal
d = decimal.Decimal('00.26598')
print("Original Number : ",d)
for i in range(1, 5):
decimal.getcontext().prec = i
print("Precision-",i, ':', d * 1)
Sample Output:
Original Number : 0.26598 Precision- 1 : 0.3 Precision- 2 : 0.27 Precision- 3 : 0.266 Precision- 4 : 0.2660
Pictorial Presentation:
Flowchart:
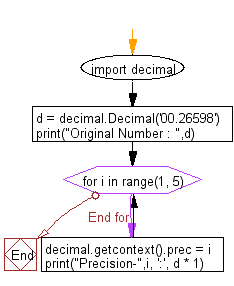
For more Practice: Solve these Related Problems:
- Write a Python program to round a specified Decimal number to various precisions (1 to 4) and print each rounded value.
- Write a Python function that takes a Decimal and a precision value, rounds the number accordingly, and returns the rounded result as a string.
- Write a Python script to prompt for a Decimal number and a desired precision, then output the number rounded to that precision using the quantize() method.
- Write a Python program to compare the output of rounding a Decimal number using different precision settings and display the differences.
Go to:
Previous: Write a Python program to retrieve the current global context (public properties) for all decimal.
Next: Write a Python program to round a specified number upwards towards infinity and down towards negative infinity of precision 4.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.