Python Math: Round a specified number upwards towards infinity and down towards negative infinity
41. Upward and Downward Rounding with Precision
Write a Python program to round a specified number upwards towards infinity and down towards negative infinity with precision 4.
Sample Solution:
Python Code:
import decimal
context = decimal.getcontext()
value = decimal.Decimal(1) / decimal.Decimal(17)
print("1/17 = ",value)
context.prec = 4
print("Precision: ",4)
context.rounding = getattr(decimal, 'ROUND_CEILING')
value = decimal.Decimal(1) / decimal.Decimal(17)
print("Round upwards towards infinity: ",value)
context.rounding = getattr(decimal, 'ROUND_FLOOR')
value = decimal.Decimal(1) / decimal.Decimal(17)
print("Round down towards negative infinity: ",value)
Sample Output:
1/17 = 0.05882352941176470588235294118 Precision: 4 Round upwards towards infinity: 0.05883 Round down towards negative infinity: 0.05882
Flowchart:
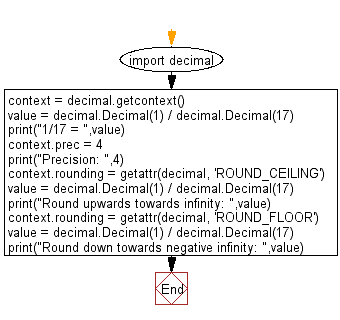
For more Practice: Solve these Related Problems:
- Write a Python program to round a given Decimal number upward (ceil) and downward (floor) with precision 4, and then print both results.
- Write a Python function that takes a Decimal and rounds it to 4 decimal places using both decimal.ROUND_CEILING and decimal.ROUND_FLOOR, then returns the two values.
- Write a Python script to demonstrate the difference between rounding up and down for several Decimal values at precision 4, printing a comparison table.
- Write a Python program to generate a list of Decimal numbers, round each upwards and downwards with precision 4, and print the original and rounded values side by side.
Go to:
Previous: Write a Python program to round a specified decimal by setting precision (between 1 and 4).
Next: Write a Python program to get the local and default precision.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.