Python Math: Convert a floating point number to an approximate rational value
47. Approximate PI as a Fraction
Write a Python program to convert a floating point number (PI) to an approximate rational value on the various denominators.
Note: max_denominator = 1000000
Sample Solution:
Python Code:
import fractions
import math
print('PI =', math.pi)
f_pi = fractions.Fraction(str(math.pi))
print('No limit =', f_pi)
for d in [1, 5, 50, 90, 100, 500, 1000000]:
limited = f_pi.limit_denominator(d)
print('{0:8} = {1}'.format(d, limited))
Sample Output:
PI = 3.141592653589793 No limit = 3141592653589793/1000000000000000 1 = 3 5 = 16/5 50 = 22/7 90 = 267/85 100 = 311/99 500 = 355/113 1000000 = 3126535/995207
Flowchart:
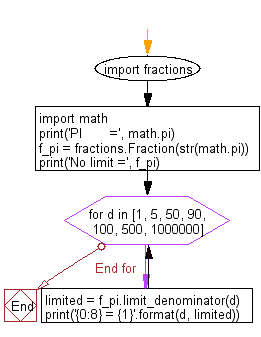
For more Practice: Solve these Related Problems:
- Write a Python program to convert the value of PI to a Fraction using a given maximum denominator and print the resulting approximation.
- Write a Python function that accepts a maximum denominator, approximates PI as a Fraction, and returns the numerator and denominator.
- Write a Python script to compute several rational approximations of PI with different maximum denominators and display them side by side.
- Write a Python program to compare the Fraction approximation of PI with the built-in math.pi and print the absolute difference.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to add, subtract, multiply and divide two fractions.
Next: Write a Python program to generate random float numbers in a specific numerical range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.