Python Math: Generate random even integers in a specific numerical range
Python Math: Exercise-50 with Solution
Write a Python program to generate random even integers in a specific numerical range.
Sample Solution:
Python Code:
import random
for x in range(6):
print(random.randrange(10, 100, 2), end=' ')
Sample Output:
74 54 12 58 18 82
Pictorial Presentation:
Flowchart:
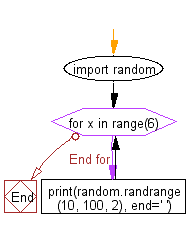
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to generate random integers in a specific numerical range.
Next: Write a Python program to get a single random element from a specified string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/math/python-math-exercise-50.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics