Python Math: Print the floating point from mantissa, exponent pair
Python Math: Exercise-58 with Solution
Write a Python program to print the floating point of the mantissa, exponent pair.
Sample Solution:
Python Code:
import math
print('{:^7} {:^8} {:^17}'.format('Mantissa', 'Exponent', 'Floating point value'))
print('{:-^8} {:-^8} {:-^20}'.format('', '', ''))
for m, e in [ (0.7, -3),
(0.3, 0),
(0.5, 3),
]:
x = math.ldexp(m, e)
print('{:7.2f} {:7d} {:7.2f}'.format(m, e, x))
Sample Output:
Mantissa Exponent Floating point value -------- -------- -------------------- 0.70 -3 0.09 0.30 0 0.30 0.50 3 4.00
Flowchart:
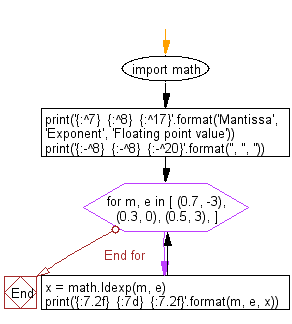
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to calculate the standard deviation of the following data.
Next: Write a Python program to split fractional and integer parts of a floating point number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/math/python-math-exercise-58.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics