Python Math: Create a simple math quiz
63. Simple Math Quiz Creator
Write a Python program to create a simple math quiz.
Sample Solution:
Python Code:
import random
#https://gist.github.com/cwil323/9b1bfd25523f75d361879adfed550be2
def display_intro():
title = "** A Simple Math Quiz **"
print("*" * len(title))
print(title)
print("*" * len(title))
def display_menu():
menu_list = ["1. Addition", "2. Subtraction", "3. Multiplication", "4. Integer Division", "5. Exit"]
print(menu_list[0])
print(menu_list[1])
print(menu_list[2])
print(menu_list[3])
print(menu_list[4])
def display_separator():
print("-" * 24)
def get_user_input():
user_input = int(input("Enter your choice: "))
while user_input > 5 or user_input <= 0:
print("Invalid menu option.")
user_input = int(input("Please try again: "))
else:
return user_input
def get_user_solution(problem):
print("Enter your answer")
print(problem, end="")
result = int(input(" = "))
return result
def check_solution(user_solution, solution, count):
if user_solution == solution:
count = count + 1
print("Correct.")
return count
else:
print("Incorrect.")
return count
def menu_option(index, count):
number_one = random.randrange(1, 21)
number_two = random.randrange(1, 21)
if index is 1:
problem = str(number_one) + " + " + str(number_two)
solution = number_one + number_two
user_solution = get_user_solution(problem)
count = check_solution(user_solution, solution, count)
return count
elif index is 2:
problem = str(number_one) + " - " + str(number_two)
solution = number_one - number_two
user_solution = get_user_solution(problem)
count = check_solution(user_solution, solution, count)
return count
elif index is 3:
problem = str(number_one) + " * " + str(number_two)
solution = number_one * number_two
user_solution = get_user_solution(problem)
count = check_solution(user_solution, solution, count)
return count
else:
problem = str(number_one) + " // " + str(number_two)
solution = number_one // number_two
user_solution = get_user_solution(problem)
count = check_solution(user_solution, solution, count)
return count
def display_result(total, correct):
if total > 0:
result = correct / total
percentage = round((result * 100), 2)
if total == 0:
percentage = 0
print("You answered", total, "questions with", correct, "correct.")
print("Your score is ", percentage, "%. Thank you.", sep = "")
def main():
display_intro()
display_menu()
display_separator()
option = get_user_input()
total = 0
correct = 0
while option != 5:
total = total + 1
correct = menu_option(option, correct)
option = get_user_input()
print("Exit the quiz.")
display_separator()
display_result(total, correct)
main()
Sample Output:
************************ ** A Simple Math Quiz ** ************************ 1. Addition 2. Subtraction 3. Multiplication 4. Integer Division 5. Exit ------------------------ Enter your choice: 1 Enter your answer 16 + 16 = 32 Correct. Enter your choice: 5 Exit the quiz. ------------------------ You answered 1 questions with 1 correct. Your score is 100.0%. Thank you.
Flowchart:
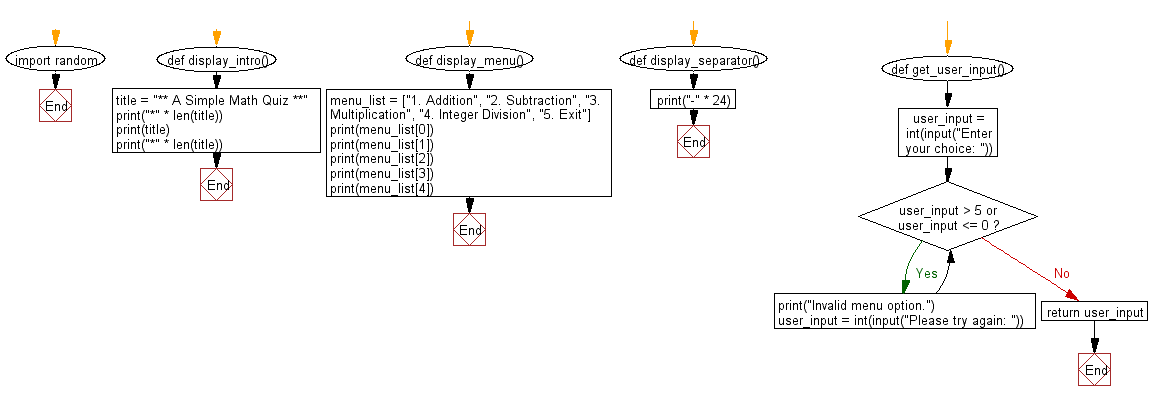
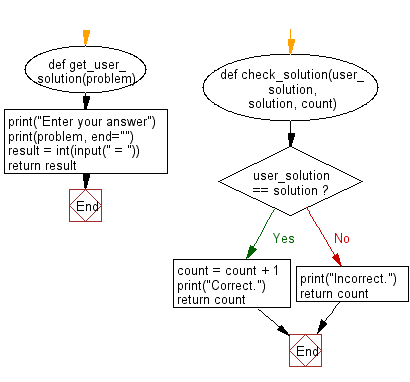
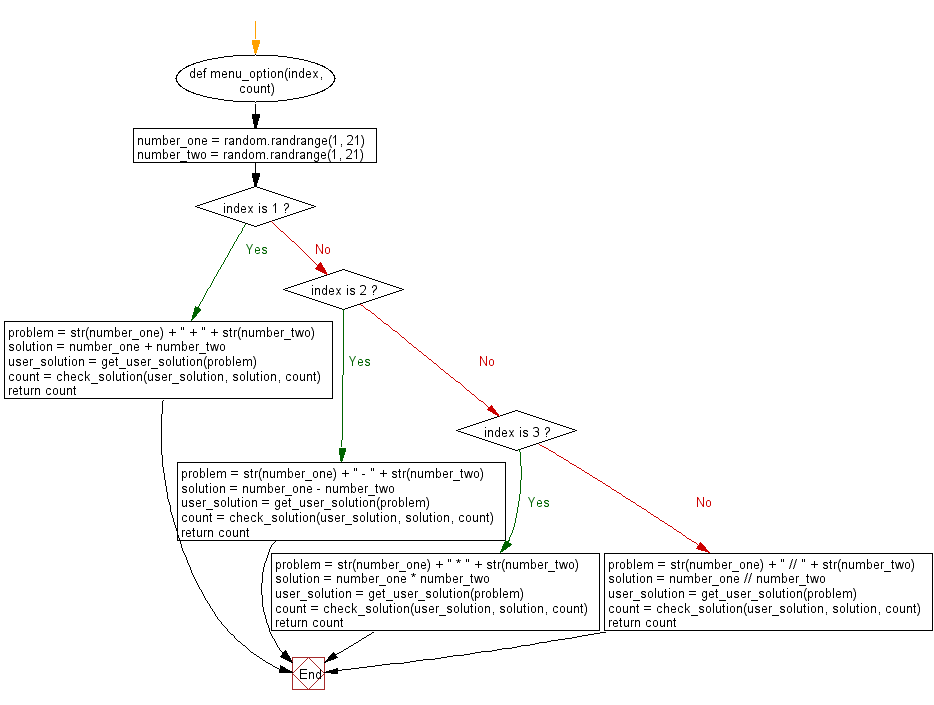
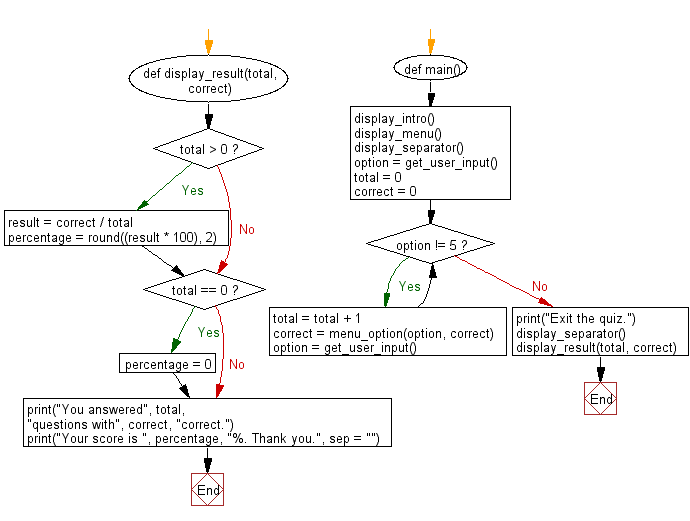
For more Practice: Solve these Related Problems:
- Write a Python program to create an interactive math quiz that asks random arithmetic questions and computes the user's score.
- Write a Python function that displays a menu for a math quiz, collects user responses, and outputs a final score as a percentage.
- Write a Python script to generate a simple math quiz with multiple choice questions and print the correct answer after each response.
- Write a Python program to implement a timed math quiz where users have a limited time to answer each question, then print the overall performance.
Go to:
Previous: Write a Python program to calculate a grid of hexagon coordinates of the given radius given lower-left and upper-right coordinates. The function will return a list of lists containing 6 tuples of x, y point coordinates. These can be used to construct valid regular hexagonal polygons.
Next: Write a Python program to calculate the volume of a tetrahedron.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.