Python Math: Calculate the discriminant value
Python Math: Exercise-9 with Solution
Write a Python program to calculate the discriminant value.
Note: The discriminant is the name given to the expression that appears under the square root (radical) sign in the quadratic formula.
Sample Solution:
Python Code:
def discriminant():
x_value = float(input('The x value: '))
y_value = float(input('The y value: '))
z_value = float(input('The z value: '))
discriminant = (y_value**2) - (4*x_value*z_value)
if discriminant > 0:
print('Two Solutions. Discriminant value is:', discriminant)
elif discriminant == 0:
print('One Solution. Discriminant value is:', discriminant)
elif discriminant < 0:
print('No Real Solutions. Discriminant value is:', discriminant)
discriminant()
Sample Output:
The x value: 4 The y value: 8 The z value: 2 Two Solutions. Discriminant value is: 32.0
Flowchart:
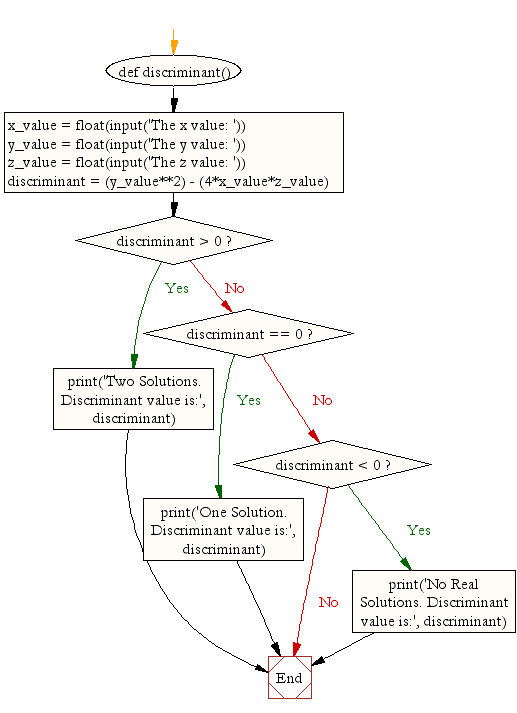
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to calculate the area of a sector.
Next: Write a Python program to find the smallest multiple of the first n
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/math/python-math-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics