Python Exercises: Sum of all prime numbers in a list of integers
94. Sum of Prime Numbers in List
From Wikipedia,
A prime number (or a prime) is a natural number greater than 1 that is not a product of two smaller natural numbers. A natural number greater than 1 that is not prime is called a composite number. For example, 5 is prime because the only ways of writing it as a product, 1 × 5 or 5 × 1, involve 5 itself. However, 4 is composite because it is a product (2 × 2) in which both numbers are smaller than 4.
Write a Python program to calculate the sum of all prime numbers in a given list of positive integers.
Sample Data:
([1, 3, 4, 7, 9]) -> 10
([]) -> Empty list!
([11, 37, 444]) -> 48
Sample Solution-1:
Python Code:
def test(nums):
if len(nums) > 0:
return sum(list(filter(lambda x: (x > 1 and all(x % y != 0 for y in range(2, x))), nums)))
return "Empty list!"
nums = [1, 3, 4, 7, 9]
print("Original list:")
print(nums)
print("Sum of all prime numbers in the said list of numbers:")
print(test(nums))
nums = []
print("\nOriginal list:")
print(nums)
print("Sum of all prime numbers in the said list of numbers:")
print(test(nums))
nums = [11, 37, 444]
print("\nOriginal list:")
print(nums)
print("Sum of all prime numbers in the said list of numbers:")
print(test(nums))
Sample Output:
Original list: [1, 3, 4, 7, 9] Sum of all prime numbers in the said list of numbers: 10 Original list: [] Sum of all prime numbers in the said list of numbers: Empty list! Original list: [11, 37, 444] Sum of all prime numbers in the said list of numbers: 48
Flowchart:
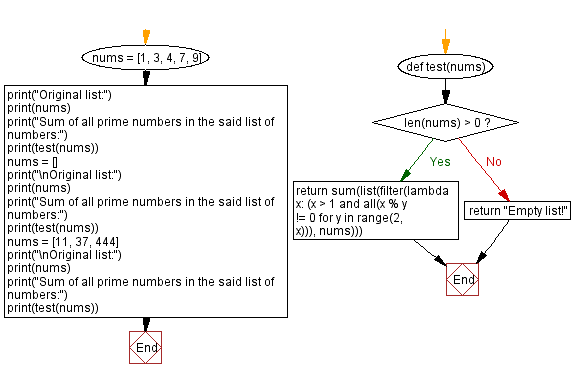
Sample Solution-2:
Python Code:
def test(nums):
if len(nums) > 0:
e = lambda a: 2 in [a, 2**a%a]
return sum(filter(e, nums))
else:
return "Empty list!"
nums = [1, 3, 4, 7, 9]
print("Original list:")
print(nums)
print("Sum of all prime numbers in the said list of numbers:")
print(test(nums))
nums = []
print("\nOriginal list:")
print(nums)
print("Sum of all prime numbers in the said list of numbers:")
print(test(nums))
nums = [11, 37, 444]
print("\nOriginal list:")
print(nums)
print("Sum of all prime numbers in the said list of numbers:")
print(test(nums))
Sample Output:
Original list: [1, 3, 4, 7, 9] Sum of all prime numbers in the said list of numbers: 10 Original list: [] Sum of all prime numbers in the said list of numbers: Empty list! Original list: [11, 37, 444] Sum of all prime numbers in the said list of numbers: 48
Flowchart:
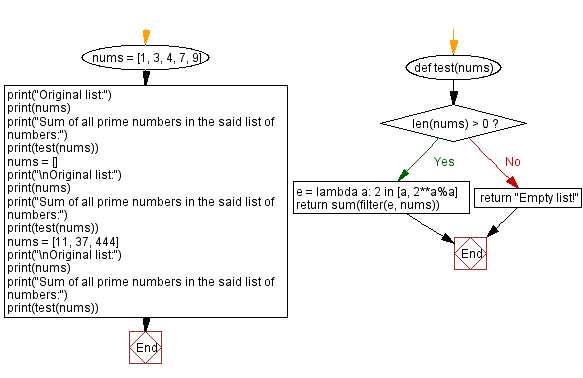
For more Practice: Solve these Related Problems:
- Write a Python program to filter a list of integers to identify prime numbers and then calculate their sum, printing the final total.
- Write a Python function that takes a list of positive integers, determines which numbers are prime, and returns the sum of these primes.
- Write a Python script to prompt the user for a list of numbers, compute the sum of the primes among them, and display the result, handling empty lists appropriately.
- Write a Python program to generate a random list of integers, extract the prime numbers using a helper function, and then output the sum of the primes.
Go to:
Previous Python Exercise: Rearrange the digits of a number.
Next Python Exercise: File Exercises Home.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.