Python: Parse a given CSV string and get the list of lists of string values
3. CSV String Parser
Write a Python program to parse a given CSV string and get a list of lists of string values. Use csv.reader
We will get a list of lists of string values after parsing a CSV formatted string where each list of strings represents a line. For example "x, y, z" will become [["x", "y", "z"]].
Sample Solution:
Python Code:
Sample Output:
Original string: 1,2,3 4,5,6 7,8,9 List of CSV formatted strings: ['1,2,3', '4,5,6', '7,8,9'] List representation of the CSV file: [['1', '2', '3'], ['4', '5', '6'], ['7', '8', '9']]
Flowchart:
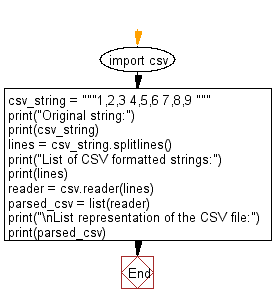
For more Practice: Solve these Related Problems:
- Write a Python program to parse a multiline CSV string using csv.reader and output the result as a list of lists.
- Write a Python function that takes a CSV formatted string with custom delimiters and returns a nested list of strings using csv.reader.
- Write a Python script to convert a CSV string with quoted fields into a list of lists, then print each sublist.
- Write a Python program to parse a CSV string containing newline characters within quoted fields and display the resulting list of lists.
Go to:
Previous: Write a Python program to count the number of lines in a given CSV file.
Next: Write a Python program to read the current line from a given CSV file.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.