Python: Generate a random color hex, alphabetical string, random value and multiple of 7
Python module: Exercise-1 with Solution
Write a Python program to generate a random color hex, a random alphabetical string, random value between two integers (inclusive) and a random multiple of 7 between 0 and 70.
Use random.randint()
Sample Solution:
Python Code:
import random
import string
print("Generate a random color hex:")
print("#{:06x}".format(random.randint(0, 0xFFFFFF)))
print("\nGenerate a random alphabetical string:")
max_length = 255
s = ""
for i in range(random.randint(1, max_length)):
s += random.choice(string.ascii_letters)
print(s)
print("Generate a random value between two integers, inclusive:")
print(random.randint(0, 10))
print(random.randint(-7, 7))
print(random.randint(1, 1))
print("Generate a random multiple of 7 between 0 and 70:")
print(random.randint(0, 10) * 7)
Sample Output:
Generate a random color hex: #eb76d4 Generate a random alphabetical string: lGhPpBDqfCgXKzSbGcnmcDWBEZeiqcUqztgvwcXfVyPslOggKdbIxOejJfFMgspqrgskanNYpscJEOVIpYkGGNxQlaqeeubGDbQSBhBedrdOyqOmKPTZvzKmKVoidsuShSCapEXxxNJRCxXOwYUUPBefKmJiidGxHwvOxAEujZGjJjTqjRtuEXgyRsPQpqlqOJJjKHAPHmIJLpMvLTRVqwSeLCIDRdMnYpbg Generate a random value between two integers, inclusive: 0 4 1 Generate a random multiple of 7 between 0 and 70: 70
Flowchart:
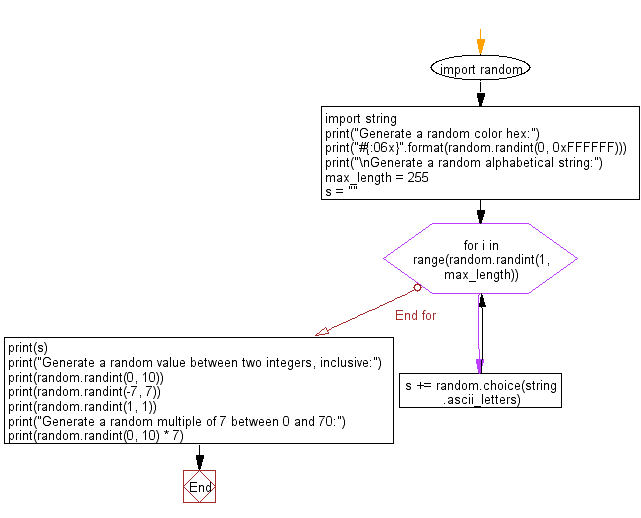
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python module methods Exercise Home.
Next: Write a Python program to select a random element from a list, set, dictionary (value) and a file from a directory.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/modules/python-module-random-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics