Python: Select a random element from a list, set, dictionary and a file from a directory
2. Random Element Selection
Write a Python program to select a random element from a list, set, dictionary-value, and file from a directory.
Use random.choice
Sample Solution:
Python Code:
import random
import os
print("Select a random element from a list:")
elements = [1, 2, 3, 4, 5]
print(random.choice(elements))
print(random.choice(elements))
print(random.choice(elements))
print("\nSelect a random element from a set:")
elements = set([1, 2, 3, 4, 5])
# convert to tuple because sets are invalid inputs
print(random.choice(tuple(elements)))
print(random.choice(tuple(elements)))
print(random.choice(tuple(elements)))
print("\nSelect a random value from a dictionary:")
d = {"a": 1, "b": 2, "c": 3, "d": 4, "e": 5}
key = random.choice(list(d))
print(d[key])
key = random.choice(list(d))
print(d[key])
key = random.choice(list(d))
print(d[key])
print("\nSelect a random file from a directory.:")
print(random.choice(os.listdir("/")))
Sample Output:
Select a random element from a list: 3 4 5 Select a random element from a set: 1 3 5 Select a random value from a dictionary: 5 4 4 Select a random file from a directory.: proc
Flowchart:
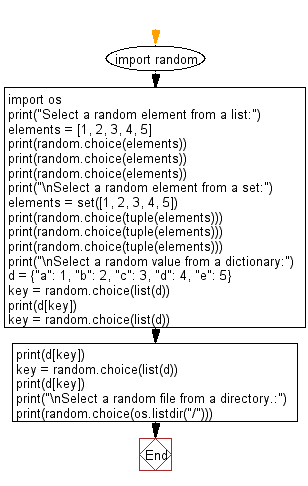
For more Practice: Solve these Related Problems:
- Write a Python program to randomly select an element from a list of file names in a directory and print the chosen file name.
- Write a Python function that picks a random value from a set of integers and a random value from a dictionary’s values, then prints both.
- Write a Python script to read all filenames from a directory into a list, then use random.choice() to select one and print its full path.
- Write a Python program that selects a random element from each of a list, a tuple, and a dictionary (by its values) and then prints them together.
Go to:
Previous: Write a Python program to generate a random color hex, a random alphabetical string, random value between two integers (inclusive) and a random multiple of 7 between 0 and 70.
Next: Write a Python program to generate a random alphabetical character, alphabetical string and alphabetical string of a fixed length.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.