Python: Generate a random alphabetical character, string and alphabetical strings of a fixed length
Python module: Exercise-3 with Solution
Write a Python program that generates random alphabetical characters, alphabetical strings, and alphabetical strings of a fixed length.
Use random.choice
Sample Solution:
Python Code:
import random
import string
print("Generate a random alphabetical character:")
print(random.choice(string.ascii_letters))
print("\nGenerate a random alphabetical string:")
max_length = 255
str1 = ""
for i in range(random.randint(1, max_length)):
str1 += random.choice(string.ascii_letters)
print(str1)
print("\nGenerate a random alphabetical string of a fixed length:")
str1 = ""
for i in range(10):
str1 += random.choice(string.ascii_letters)
print(str1)
Sample Output:
Generate a random alphabetical character: e Generate a random alphabetical string: wxEGKdCBCkJZrFscEDXhxAovbTkPzlfCxRQCMbuvquFXyFHivyEeqNGzeWxlKZiFzVIuyKLEtPJHbvqQTpIJoMTPFUbrQjGEfXRTlQNKviduRaNDdtEbExXhdbLKiIprgdYTivZDFjk Generate a random alphabetical string of a fixed length: ijdvKiSWwO
Flowchart:
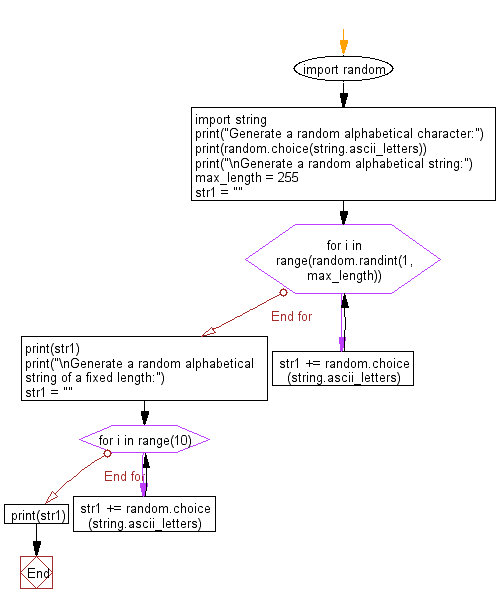
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to select a random element from a list, set, dictionary (value) and a file from a directory.
Next: Write a Python program to construct a seeded random number generator, also generate a float between 0 and 1, excluding 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/modules/python-module-random-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics