Python: Shuffle the elements of a given list
Write a Python program to shuffle the elements of a given list.
Use random.shuffle()
Sample Solution:
Python Code:
import random
nums = [1, 2, 3, 4, 5]
print("Original list:")
print(nums)
random.shuffle(nums)
print("Shuffle list:")
print(nums)
words = ['red', 'black', 'green', 'blue']
print("\nOriginal list:")
print(words)
random.shuffle(words)
print("Shuffle list:")
print(words)
Sample Output:
Original list: [1, 2, 3, 4, 5] Shuffle list: [4, 2, 1, 5, 3] Original list: ['red', 'black', 'green', 'blue'] Shuffle list: ['green', 'blue', 'black', 'red']
Flowchart:
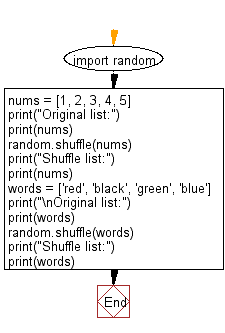
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to generate a random integer between 0 and 6 - excluding 6, random integer between 5 and 10 - excluding 10, random integer between 0 and 10, with a step of 3 and random date between two dates.
Next: Write a Python program to generate a float between 0 and 1, inclusive and generate a random float within a specific range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics