Python: Create a list of random integers and select multiple items from the said list
Write a Python program to create a list of random integers and randomly select multiple items from the said list.
Use random.sample
Sample Solution:
Python Code:
import random
print("Create a list of random integers:")
population = range(0, 100)
nums_list = random.sample(population, 10)
print(nums_list)
no_elements = 4
print("\nRandomly select",no_elements,"multiple items from the said list:")
result_elements = random.sample(nums_list, no_elements)
print(result_elements)
no_elements = 8
print("\nRandomly select",no_elements,"multiple items from the said list:")
result_elements = random.sample(nums_list, no_elements)
print(result_elements)
Sample Output:
Create a list of random integers: [46, 4, 45, 50, 43, 85, 74, 42, 99, 7] Randomly select 4 multiple items from the said list: [99, 45, 85, 50] Randomly select 8 multiple items from the said list: [46, 43, 74, 4, 45, 85, 99, 50]
Flowchart:
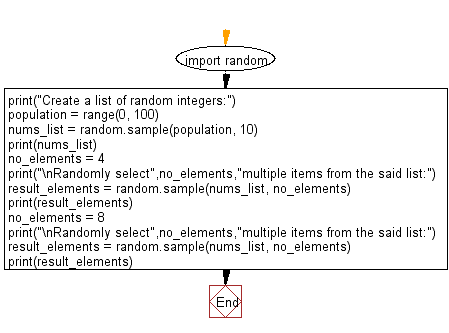
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to generate a float between 0 and 1, inclusive and generate a random float within a specific range.
Next: Write a Python program to set a random seed and get a random number between 0 and 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics