NumPy: Create a 3x3 identity matrix
Create 3x3 Identity Matrix
Write a NumPy program to create a 3x3 identity matrix.
This NumPy program generates a 3x3 identity matrix, which is a square matrix with ones on the main diagonal and zeros elsewhere. By using NumPy's built-in functions, it efficiently constructs this matrix. This program exemplifies how to create standard mathematical matrices in a straightforward manner using NumPy.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 3x3 identity matrix using np.identity()
array_2D = np.identity(3)
# Printing a message indicating a 3x3 matrix
print('3x3 matrix:')
# Printing the 3x3 identity matrix
print(array_2D)
Sample Output:
3x3 matrix: [[ 1. 0. 0.] [ 0. 1. 0.] [ 0. 0. 1.]]
Explanation:
In the above code np.identity() function creates a 2D identity matrix with a size of 3x3. An identity matrix is a square matrix with ones on the diagonal and zeros elsewhere.
Visual Presentation:
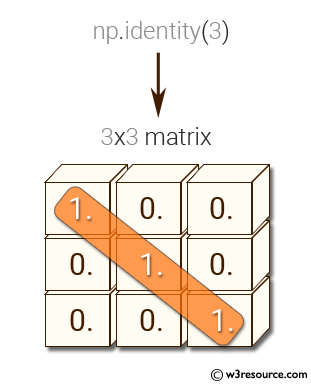
For more Practice: Solve these Related Problems:
- Generate a 4x4 identity matrix using np.eye and validate the diagonal elements.
- Create an identity matrix and then modify one of its diagonal elements.
- Construct an identity matrix with an offset diagonal using np.eye with k parameter.
- Use np.identity to create a 3x3 matrix and compute its determinant.
Go to:
PREV : Create Array of Even Integers 30 to 70
NEXT : Generate Random Number [0,1]
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.