NumPy: Create a 3X4 array using and iterate over it
Create and Iterate 3x4 Array
Write a NumPy program to create a 3X4 array and iterate over it.
This NumPy program creates a 3x4 array and then iterates over its elements. By utilizing NumPy's array creation functions, it constructs the array, and with the help of iteration techniques, it accesses and processes each element individually.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'a' containing integers from 10 to 21 and reshaping it into a 3x4 matrix using np.arange() and .reshape()
a = np.arange(10, 22).reshape((3, 4))
# Printing a message indicating the original array 'a'
print("Original array:")
print(a)
# Printing a message indicating each element of the array using np.nditer() to iterate through the elements
print("Each element of the array is:")
for x in np.nditer(a):
print(x, end=" ")
Output:
Original array: [[10 11 12 13] [14 15 16 17] [18 19 20 21]] Each element of the array is: 10 11 12 13 14 15 16 17 18 19 20 21
Explanation:
In the above code np.arange(10,22).reshape((3, 4)) creates a NumPy array containing integers from 10 to 21 using the np.arange() function. It then reshapes the array into a 3x4 matrix using the reshape() method.
for x in np.nditer(a):: This line starts a for loop that iterates over each element in the array 'a' using the np.nditer() function.
Finally print(x, end=" ") prints the current array element (x) followed by a space. The end=" " parameter ensures that the printed elements are separated by a space, rather than the default newline character.
Output: 10 11 12 13 14 15 16 17 18 19 20 21
Visual Presentation:
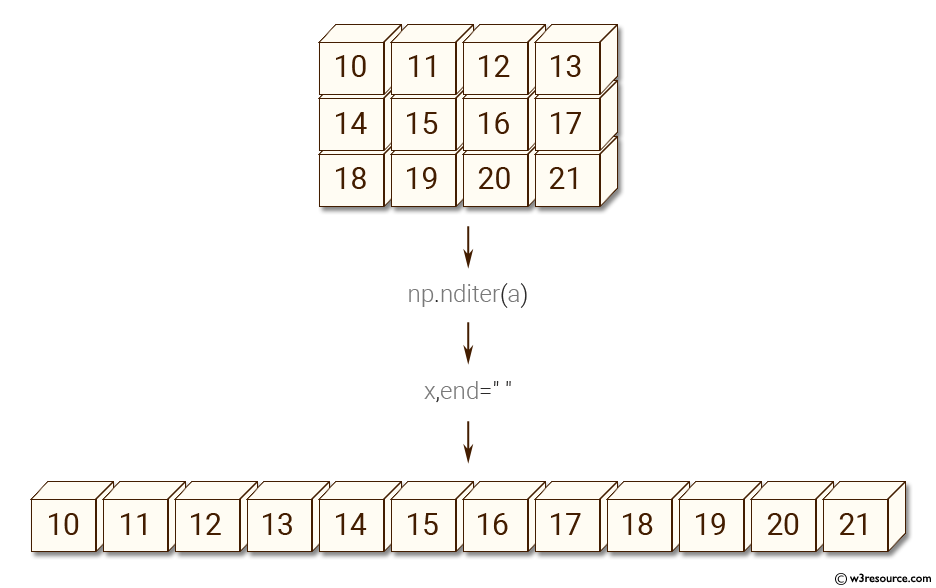
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics