NumPy: Create a vector of length 5 filled with arbitrary integers from 0 to 10
Vector of Random Integers [0,10]
Write a NumPy program to create a vector of length 5 filled with arbitrary integers from 0 to 10.
This problem involves writing a NumPy program to generate a vector of length 5 containing random integers between 0 and 10. The task requires utilizing NumPy's random number generation capabilities to create the vector efficiently while ensuring that the integers fall within the specified range.
Sample Solution :
Python Code :
Output:
Vector of length 5 filled with arbitrary integers from 0 to 10: [ 0 10 2 0 6]
Explanation:
In the above code np.random.randint() function generates an array of random integers. The function takes three arguments: the lower bound (inclusive), the upper bound (exclusive), and the number of random integers to generate. In this case, it generates an array of 5 random integers between 0 (inclusive) and 11 (exclusive).
Visual Presentation:
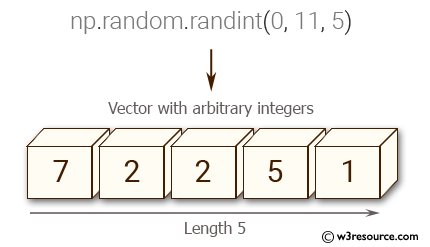
For more Practice: Solve these Related Problems:
- Generate a vector of 5 random integers between 0 and 10 using np.random.randint.
- Create an array of random integers and ensure that all elements are unique.
- Construct a vector of random integers and sort the resulting array in ascending order.
- Generate a random vector of integers and compute its frequency distribution.
Go to:
PREV : Change Sign of Range 9-15
NEXT : Multiply Two Vectors
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.