NumPy: Create a 3x3x3 array filled with arbitrary values
Create 3x3x3 Array of Values
Write a NumPy program to create a 3x3x3 array filled with arbitrary values.
This problem involves writing a NumPy program to generate a 3x3x3 array filled with arbitrary values. The task requires utilizing NumPy's array creation functionalities to efficiently construct the multi-dimensional array with arbitrary values. By specifying the desired shape of the array and providing arbitrary values, the program generates a 3x3x3 array suitable for various computational and analytical tasks.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 3x3x3 array filled with random numbers between 0 and 1 using np.random.random()
x = np.random.random((3, 3, 3))
# Printing the randomly generated 3x3x3 array 'x'
print(x)
Output:
[[[ 0.51919099 0.31268732 0.58506582] [ 0.12730206 0.30556451 0.55981097] [ 0.92910493 0.73947119 0.14252086]] [[ 0.96159407 0.21341612 0.6814465 ] [ 0.71884351 0.01271011 0.98812225] [ 0.47553515 0.04584955 0.59425412]] [[ 0.44064227 0.26612916 0.58861619] [ 0.37935141 0.0640071 0.53717733] [ 0.77986385 0.0771148 0.92183522]]]
Explanation:
The above code creates a 3D array of random numbers between 0 and 1 with the shape (3, 3, 3) and prints the resulting array.
Here ‘np.random.random((3, 3, 3))’ creates a 3D array with the shape (3, 3, 3), filled with random numbers between 0 and 1. The input to np.random.random() is a tuple representing the desired shape of the output array.
Finally print(x) prints the generated 3D array to the console.
Visual Presentation:
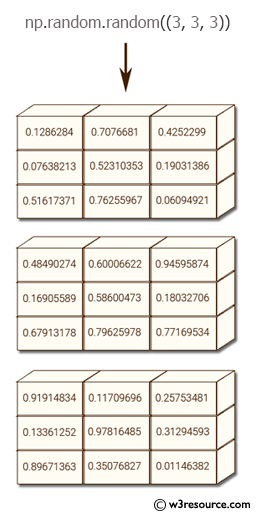
For more Practice: Solve these Related Problems:
- Construct a 3x3x3 array with sequential integers and verify its reshaped structure.
- Generate a 3x3x3 array and compute the sum along each of its three axes.
- Create a 3x3x3 array filled with a constant value, then modify a specific 2D slice.
- Form a 3x3x3 array and extract the central 2D subarray from each of its matrices.
Go to:
PREV : Staggered 4x4 Matrix (0,1)
NEXT : Compute Sums (All, Row, Column)
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.