NumPy: Compute sum of all elements, sum of each column and sum of each row of a given array
Compute Sums (All, Row, Column)
Write a NumPy program to compute the sum of all elements, the sum of each column and the sum of each row in a given array.
This problem involves writing a NumPy program to compute the sum of all elements, as well as the sum of each column and each row in a given array. The task requires leveraging NumPy's array manipulation functions to efficiently calculate these sums. By utilizing NumPy's built-in functions such as np.sum along appropriate axes, the program extracts the sums of rows, columns, and the entire array, providing comprehensive insights into the array's data distribution.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'x' with a 2x2 shape
x = np.array([[0, 1], [2, 3]])
# Printing a message indicating the original array 'x'
print("Original array:")
print(x)
# Calculating and printing the sum of all elements in the array 'x' using np.sum()
print("Sum of all elements:")
print(np.sum(x))
# Calculating and printing the sum of each column in the array 'x' using np.sum() with axis=0
print("Sum of each column:")
print(np.sum(x, axis=0))
# Calculating and printing the sum of each row in the array 'x' using np.sum() with axis=1
print("Sum of each row:")
print(np.sum(x, axis=1))
Output:
Original array: [[0 1] [2 3]] Sum of all elements: 6 Sum of each column: [2 4] Sum of each row: [1 5]
Explanation:
In the above code -
‘x = np.array([[0, 1], [2, 3]])’ creates a 2D array 'x' with the shape (2, 2) and elements [[0, 1], [2, 3]].
In the ‘print(np.sum(x))’ statement, np.sum() function is used to calculate the sum of all elements in the array 'x' and prints the result. In this case, the sum is 0+1+2+3 = 6.
In the ‘print(np.sum(x, axis=0))’ statement, np.sum() function is used to calculate the sum of the elements in the array 'x' along the first axis (axis 0, corresponding to columns). The result is a 1D array with the sum of each column: [0+2, 1+3] = [2, 4]. The output is then printed.
Finally in ‘print(np.sum(x, axis=1))’ statement np.sum() function calculates the sum of the elements in the array 'x' along the second axis (axis 1, corresponding to rows). The result is a 1D array with the sum of each row: [0+1, 2+3] = [1, 5]. The output is then printed.
Visual Presentation:
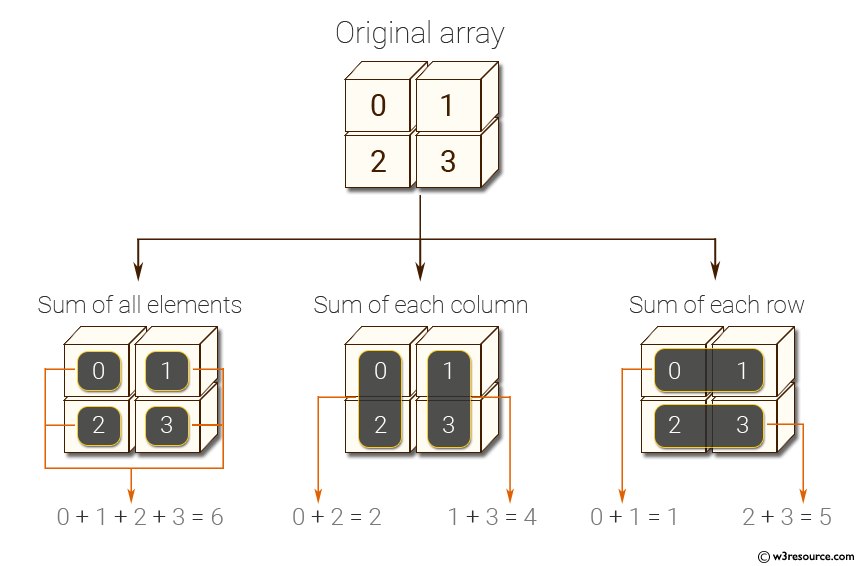
Python-Numpy Code Editor:
Previous: NumPy program to create a 3x3x3 array filled with arbitrary values.Next: NumPy program to compute the inner product of two given vectors.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics