NumPy: Save a given array to a text file and load it
Save and Load Array from Text File
Write a NumPy program to save a given array to a text file and load it.
This problem involves writing a NumPy program to save a given array to a text file and subsequently load it back into the program. The task requires utilizing NumPy's "savetxt()" function to store the array data in a human-readable text format and the "loadtxt()" function to read the data back into a NumPy array. This process demonstrates how to manage array data using text files for storage and retrieval.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Importing the 'os' module for operating system-dependent functionality
import os
# Creating a NumPy array 'x' with integers from 0 to 11 and reshaping it to a 4x3 matrix using np.arange() and .reshape()
x = np.arange(12).reshape(4, 3)
# Printing a message indicating the original array 'x'
print("Original array:")
print(x)
# Creating a string 'header' containing column names 'col1 col2 col3'
header = 'col1 col2 col3'
# Saving the array 'x' into a text file named 'temp.txt' using np.savetxt()
# Specifying the format as "%d" (integer) and including a header with column names
np.savetxt('temp.txt', x, fmt="%d", header=header)
# Printing a message indicating the content of the text file after loading
print("After loading, content of the text file:")
# Loading the data from 'temp.txt' into the variable 'result' using np.loadtxt()
result = np.loadtxt('temp.txt')
# Printing the loaded data 'result' from the text file
print(result)
Output:
Original array: [[ 0 1 2] [ 3 4 5] [ 6 7 8] [ 9 10 11]] After loading, content of the text file: [[ 0. 1. 2.] [ 3. 4. 5.] [ 6. 7. 8.] [ 9. 10. 11.]]
Explanation:
In the above code -
The ‘x = np.arange(12).reshape(4, 3)’ statement creates a 1D array with elements ranging from 0 to 11, then reshapes it into a 2D array 'x' of shape (4, 3).
‘header = 'col1 col2 col3' statement creates a string 'header' that will be used as the header for the text file.
‘np.savetxt('temp.txt', x, fmt="%d", header=header)’ statement saves the 2D NumPy array 'x' to a text file named 'temp.txt' using the integer format ("%d") for the elements, and adds the specified header to the file.
‘result = np.loadtxt('temp.txt')’ statement loads the contents of the 'temp.txt' file into a NumPy array named 'result'.
Finally print(result) prints the loaded 'result' array.
Visual Presentation:
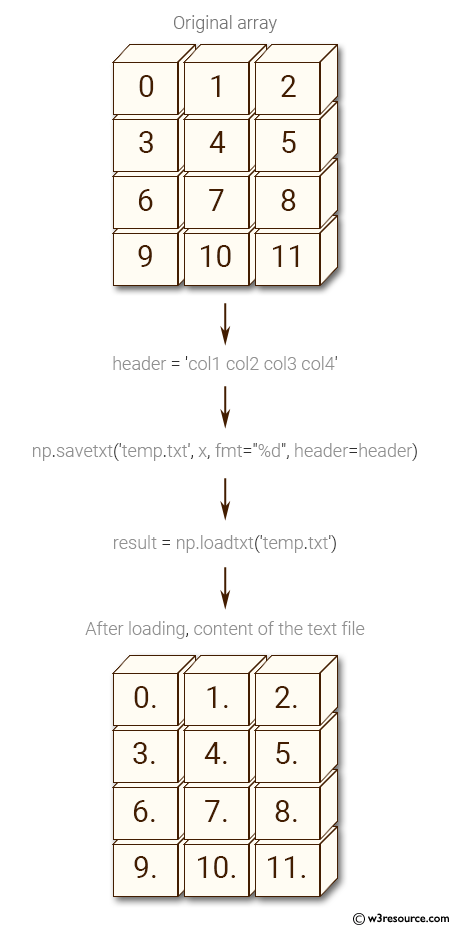
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics