NumPy: Test whether any of the elements of a given array is non-zero
Test Any Non-Zero
Write a NumPy program to test if any of the elements of a given array are non-zero.
This problem involves using NumPy to perform a check on the elements of an array. The task is to write a program that determines if any of the elements in a given NumPy array are non-zero. This is useful for situations where the presence of non-zero values is significant, such as checking for valid data entries or detecting activity in datasets.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'x' containing elements 1, 0, 0, and 0
x = np.array([1, 0, 0, 0])
# Printing the original array 'x'
print("Original array:")
print(x)
# Checking if any element in the array 'x' is non-zero and printing the result
print("Test whether any of the elements of a given array is non-zero:")
print(np.any(x))
# Reassigning array 'x' to a new array containing all elements as zero
x = np.array([0, 0, 0, 0])
# Printing the new array 'x'
print("Original array:")
print(x)
# Checking if any element in the updated array 'x' is non-zero and printing the result
print("Test whether any of the elements of a given array is non-zero:")
print(np.any(x))
Output:
Original array: [1 0 0 0] Test whether any of the elements of a given array is non-zero: True Original array: [0 0 0 0] Test whether any of the elements of a given array is non-zero: False
Explanation:
In the above code –
x = np.array([1, 0, 0, 0]): This line creates a NumPy array 'x' with the elements 1, 0, 0, and 0.
print(np.any(x)): This line uses the np.any() function to test if any elements in the array 'x' are non-zero (i.e., not equal to zero). In this case, the first element is non-zero, so the function returns True.
x = np.array([0, 0, 0, 0]): This line creates a new NumPy array 'x' with all elements equal to 0.
print(np.any(x)): This line uses the np.any() function again to test if any elements in the new array 'x' are non-zero. In this case, all elements are zero, so the function returns False.
Visual Presentation:
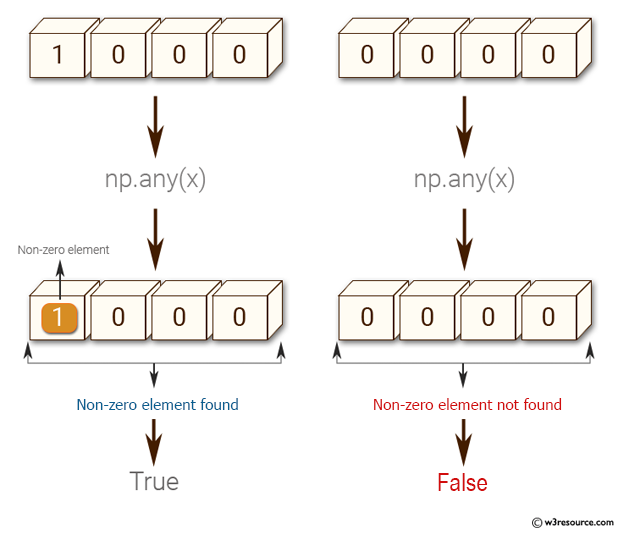
For more Practice: Solve these Related Problems:
- Determine if any element in a flattened array is non-zero without using np.any.
- Write a function that checks for at least one non-zero in an array of mixed types.
- Test a multi-dimensional array for any non-zero entries using array flattening and logical checks.
- Use boolean indexing to sum up non-zero elements and decide if any exist.
Go to:
PREV : Test None are Zero
NEXT : Test Finiteness of Elements
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.