NumPy: Test element-wise for positive or negative infinity.
Test for Infinity
Write a NumPy program to test elements-wise for positive or negative infinity.
This problem involves using NumPy to check each element in an array for positive or negative infinity. The task is to write a program that tests whether elements in a given NumPy array are either positive infinity or negative infinity. This is useful for data validation, cleaning, and preprocessing steps where infinite values might indicate errors or special cases in the dataset. The program demonstrates how to leverage NumPy's functions to handle and identify infinite values effectively.
Sample Solution :
Python Code :
# Import the NumPy library
import numpy as np
# Create a sample NumPy array with various values including positive and negative infinity
array = np.array([1, -2, np.inf, -np.inf, 0, 3.5])
# Test each element for positive or negative infinity
result_pos_inf = np.isposinf(array)
result_neg_inf = np.isneginf(array)
# Print the results
print("Element-wise positive infinity check:", result_pos_inf)
print("Element-wise negative infinity check:", result_neg_inf)
Output:
Element-wise positive infinity check: [False False True False False False] Element-wise negative infinity check: [False False False True False False]
Explanation:
In the above code –
The code begins by importing the NumPy library, which is essential for numerical operations on arrays. It then creates a sample NumPy array that includes a mix of finite numbers, positive infinity ('np.inf'), and negative infinity ('-np.inf'). The code uses the "np.isposinf()" function to test each element in the array for positive infinity and stores the result in 'result_pos_inf'. Similarly, it uses the "np.isneginf()" function to check for negative infinity, storing the result in 'result_neg_inf'. Finally, it prints the results of these element-wise checks, showing which elements are positive or negative infinity.
Visual Presentation:
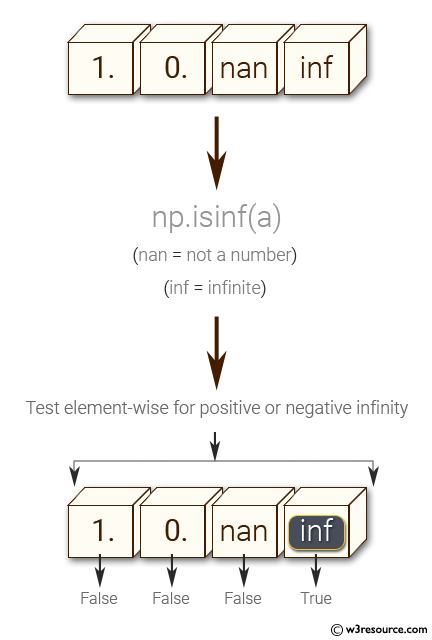
For more Practice: Solve these Related Problems:
- Identify the indices where elements in an array are either positive or negative infinity.
- Replace infinite values in a given array with the maximum finite value present.
- Check if an entire array is composed solely of infinite values.
- Generate a random array with potential infinities and count the infinite elements.
Python-Numpy Code Editor:
Previous: Test a given array element-wise for finiteness (not infinity or not a Number).Next: Test element-wise for NaN of a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.