NumPy: Test element-wise for NaN of a given array
Test for NaN
Write a NumPy program to test element-wise for NaN of a given array.
This problem involves using NumPy to identify elements in an array that are NaN (Not a Number). The task is to write a program that checks each element of a given NumPy array to determine if it is NaN. This is important for data validation and cleaning, as NaN values can indicate missing or undefined data that may need to be handled or removed before performing further analysis. The program demonstrates how to use NumPy's functions to effectively detect NaN values within an array.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'a' containing elements 1, 0, NaN (Not a Number), and infinity
a = np.array([1, 0, np.nan, np.inf])
# Printing the original array 'a'
print("Original array")
print(a)
# Testing the given array 'a' element-wise for NaN (Not a Number) and printing the result
print("Test element-wise for NaN:")
print(np.isnan(a))
Output:
Original array [ 1. 0. nan inf] Test element-wise for NaN: [False False True False]
Explanation:
In the above code -
np.array([1, 0, np.nan, np.inf]) creates a NumPy array 'a' with the elements 1, 0, NaN (Not a Number), and Inf (Infinity).
print(np.isnan(a)): The np.isnan() function tests element-wise if the values in the array 'a' are NaN. The function returns a boolean array of the same shape as 'a', with True for NaN values and False for other values (1, 0, and Inf).
Visual Presentation:
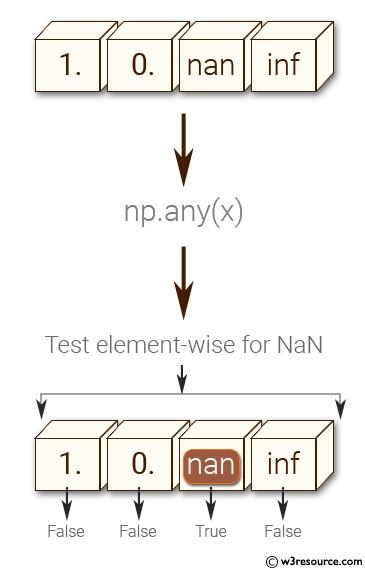
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics