NumPy: Test element-wise for complex number, real number of a given array. Also test whether a given number is a scalar type or not
NumPy: Basic Exercise-8 with Solution
Write a NumPy program to test element-wise for complex numbers, real numbers in a given array. Also test if a given number is of a scalar type or not.
This problem involves using NumPy to perform type checks on elements of an array and individual numbers. The task is to write a program that tests each element of a given NumPy array to determine if it is a complex number or a real number. Additionally, the program should check if a given number is of a scalar type. These checks are useful for data validation and type-specific operations, ensuring that numerical data is appropriately classified before performing further computations.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'a' containing various types of numbers (complex, real, scalar)
a = np.array([1+1j, 1+0j, 4.5, 3, 2, 2j])
# Printing the original array 'a'
print("Original array")
print(a)
# Checking the given array 'a' element-wise for complex numbers and printing the result
print("Checking for complex number:")
print(np.iscomplex(a))
# Checking the given array 'a' element-wise for real numbers and printing the result
print("Checking for real number:")
print(np.isreal(a))
# Checking if a value is a scalar or not (in this case, checking if 3.1 is a scalar)
print("Checking for scalar type:")
print(np.isscalar(3.1))
# Checking if a list ([3.1]) is a scalar or not (in this case, it's not a scalar)
print(np.isscalar([3.1]))
Output:
Original array [ 1.0+1.j 1.0+0.j 4.5+0.j 3.0+0.j 2.0+0.j 0.0+2.j] Checking for complex number: [ True False False False False True] Checking for real number: [False True True True True False] Checking for scalar type: True False
Explanation:
In the above exercise -
np.array([1+1j, 1+0j, 4.5, 3, 2, 2j]) creates a NumPy array 'a' with a mix of complex and real numbers.
print(np.isreal(a)): Here np.isreal() function tests element-wise if the values in the array 'a' are real numbers. The function returns a boolean array of the same shape as 'a', with True for real values and False for complex values
print(np.isscalar(3.1)): Here np.isscalar() function checks if the given object (3.1) is a scalar. In this case, 3.1 is a scalar (a single number), so the function returns True.
print(np.isscalar([3.1])): Here np.isscalar() function checks if the given object ([3.1]) is a scalar. In this case, [3.1] is a list containing a single number, but it is not a scalar, so the function returns False.
Visual Presentation:
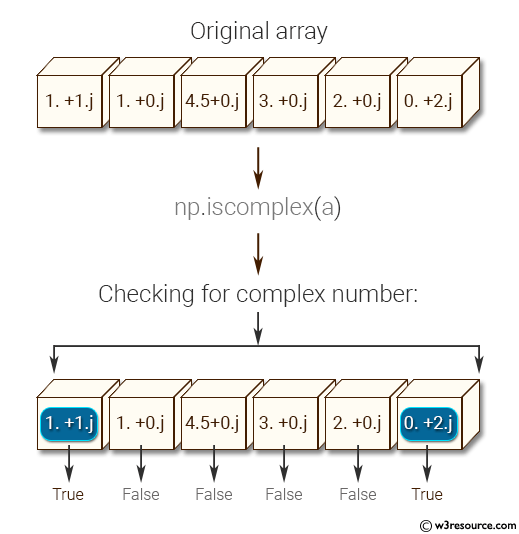
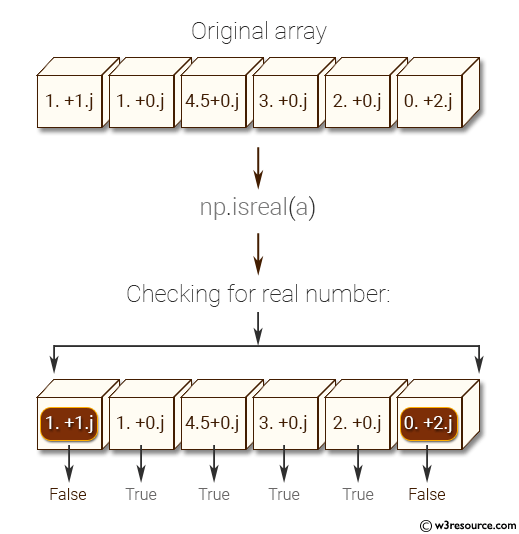
Python-Numpy Code Editor:
Previous: Test element-wise for NaN of a given array.
Next: Test if two arrays are element-wise equal within a tolerance.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/numpy/basic/numpy-basic-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics