NumPy: Compute the multiplication of two given matrixes
Write a NumPy program to compute the multiplication of two given matrixes.
Sample Matrix:
[[1, 0], [0, 1]] [[1, 2], [3, 4]]
Matrix multiplication, and the detailed calculation:
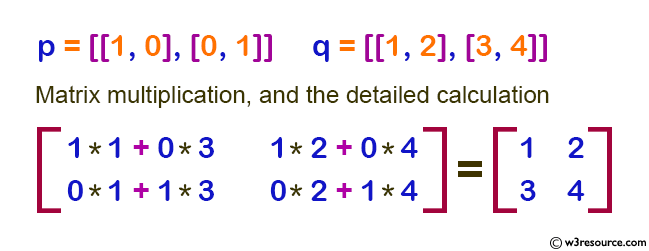
Sample Solution :
Python Code :
import numpy as np
# Define two 2x2 matrices 'p' and 'q'
p = [[1, 0], [0, 1]]
q = [[1, 2], [3, 4]]
# Display the original matrices 'p' and 'q'
print("Original matrices:")
print(p)
print(q)
# Perform matrix multiplication using np.dot
result1 = np.dot(p, q)
# Display the result of the matrix multiplication
print("Result of the matrix multiplication:")
print(result1)
Sample Output:
original matrix: [[1, 0], [0, 1]] [[1, 2], [3, 4]] Result of the said matrix multiplication: [[1 2] [3 4]]
Explanation:
p = [[1, 0], [0, 1]]
q =
is calculated as follows:
result1[0,0] = (p[0,0] * q[0,0]) + (p[0,1] * q[1,0]) = (1 * 1) + (0 * 3) = 1
result1[0,1] = (p[0,0] * q[0,1]) + (p[0,1] * q[1,1]) = (1 * 2) + (0 * 4) = 2
result1[1,0] = (p[1,0] * q[0,0]) + (p[[[1, 2], [3, 4]]
At first two 2x2 matrixes p and q have been declared.
result1 = np.dot(p, q) This code calculates the matrix multiplication (dot product) of p and q. The dot product 1,1] * q[1,0]) = (0 * 1) + (1 * 3) = 3
result1[1,1] = (p[1,0] * q[0,1]) + (p[1,1] * q[1,1]) = (0 * 2) + (1 * 4) = 4
Finally print() function prints the resulting 2x2 matrix.
.Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics