NumPy: Compute the determinant of an array
11. Determinant of an Array
Write a NumPy program to compute the determinant of an array.
From Wikipedia: In linear algebra, the determinant is a value that can be computed from the elements of a square matrix. The determinant of a matrix A is denoted det(A), det A, or |A|. Geometrically, it can be viewed as the scaling factor of the linear transformation described by the matrix.
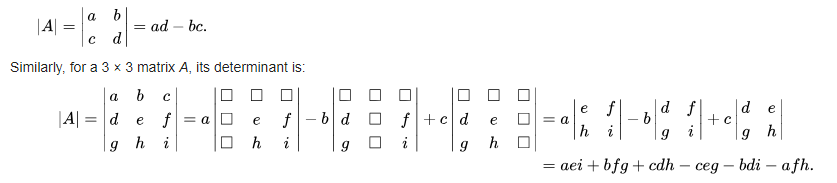
Sample Solution :
Python Code :
# Import the NumPy library and alias it as 'np'
import numpy as np
# Create a 2x2 NumPy array 'a' containing specific values
a = np.array([[1,2],[3,4]])
# Display the original array 'a'
print("Original array:")
print(a)
# Calculate the determinant of the array 'a' using np.linalg.det() function
result = np.linalg.det(a)
# Display the determinant of the array 'a'
print("Determinant of the said array:")
print(result)
Sample Output:
Original array: [[1 2] [3 4]] Determinant of the said array: -2.0000000000000004
Explanation:
In the above example –
a = np.array([[1,2],[3,4]]): This statement creates a 2x2 NumPy array a with the specified elements.
result = np.linalg.det(a): This statement computes the determinant of the matrix a. The determinant is a scalar value that can be computed from the elements of a square matrix and has important properties in linear algebra. For a 2x2 matrix [[a, b], [c, d]], the determinant is calculated as ad - bc. In this case, the determinant of a is (1*4) - (2*3) = 4 - 6 = -2.
For more Practice: Solve these Related Problems:
- Compute the determinant of a matrix with np.linalg.det and cross-check with manual diagonal multiplication for triangular matrices.
- Test the determinant function on matrices with integer elements and verify type consistency with floating-point outputs.
- Create a function that computes the determinant of a block-diagonal matrix using the product of its block determinants.
- Validate the determinant computation on a perturbed identity matrix to observe sensitivity to small changes.
Python-Numpy Code Editor:
Previous: Write a NumPy program to find a matrix or vector norm.Next: Write a NumPy program to compute the inverse of a given matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.