NumPy: Find the union of two arrays
NumPy: Array Object Exercise-22 with Solution
Write a NumPy program to find the union of two arrays. Union will return a unique, sorted array of values in each of the two input arrays.
Pictorial Presentation:
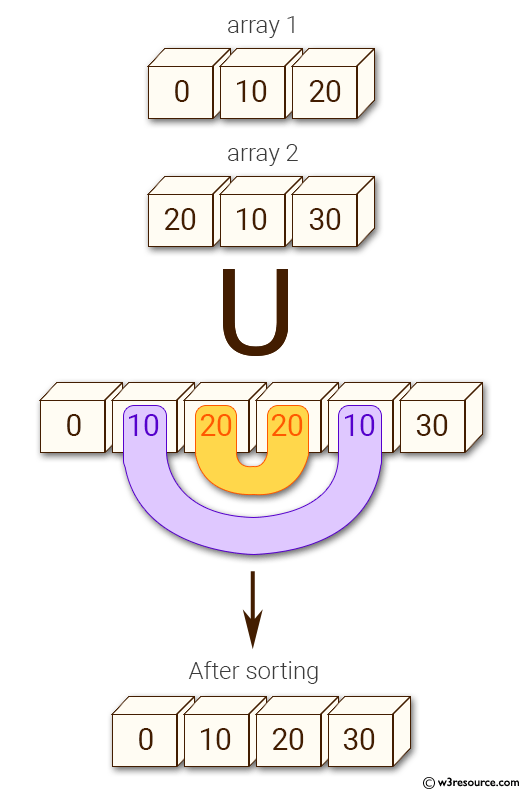
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'array1'
array1 = np.array([0, 10, 20, 40, 60, 80])
# Printing the contents of 'array1'
print("Array1: ", array1)
# Creating a Python list 'array2'
array2 = [10, 30, 40, 50, 70]
# Printing the contents of 'array2'
print("Array2: ", array2)
# Finding and printing the unique values that are in only one (not both) of the input arrays
print("Unique values that are in only one (not both) of the input arrays:")
print(np.setxor1d(array1, array2))
Sample Output:
Array1: [ 0 10 20 40 60 80] Array2: [10, 30, 40, 50, 70] Unique sorted array of values that are in either of the two input array s: [ 0 10 20 30 40 50 60 70 80]
Explanation:
In the above code –
array1 = np.array([0, 10, 20, 40, 60, 80]): Creates a NumPy array with elements 0, 10, 20, 40, 60, and 80.
array2 = [10, 30, 40, 50, 70]: Creates a Python list with elements 10, 30, 40, 50, and 70.
print(np.union1d(array1, array2)): The np.union1d function returns the sorted union of the two input arrays, which consists of all unique elements from both ‘array1’ and ‘array2’. In this case, the union is [0, 10, 20, 30, 40, 50, 60, 70, 80], so the output will be [ 0 10 20 30 40 50 60 70 80].
Python-Numpy Code Editor:
Previous: Write a NumPy program to find the set exclusive-or of two arrays. Set exclusive-or will return the sorted, unique values that are in only one (not both) of the input arrays.
Next: Write a NumPy program to test if all elements in an array evaluate to True.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/numpy/python-numpy-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics