NumPy: Get the values and indices of the elements that are bigger than 10 in a given array
Elements >10 & Their Indices
Write a NumPy program to get the values and indices of the elements that are bigger than 10 in a given array.
Pictorial Presentation:
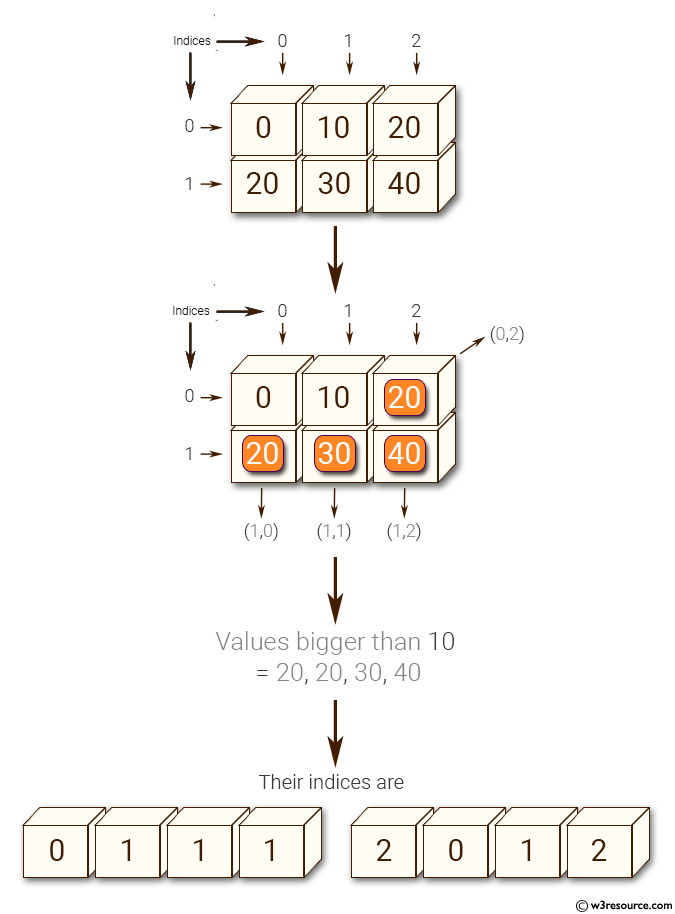
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array
x = np.array([[0, 10, 20], [20, 30, 40]])
# Displaying the original array
print("Original array: ")
print(x)
# Filtering and displaying values in the array that are greater than 10
print("Values bigger than 10 =", x[x > 10])
# Displaying indices of elements that are greater than 10 using np.nonzero()
print("Their indices are ", np.nonzero(x > 10))
Sample Output:
Original array: [[ 0 10 20] [20 30 40]] Values bigger than 10 = [20 20 30 40] Their indices are (array([0, 1, 1, 1]), array([2, 0, 1, 2]))
Explanation:
In the above code –
x = np.array([[0, 10, 20], [20, 30, 40]]): x is a 2D NumPy array with two rows and three columns.
print("Values bigger than 10 =", x[x>10]): This line prints the extracted values that are greater than 10.
print("Their indices are ", np.nonzero(x > 10)): This line prints the indices of the values greater than 10 in the array x.
For more Practice: Solve these Related Problems:
- Identify elements in a 2D array that are greater than 10 using np.where and validate the returned indices.
- Create a function that prints both the values and their indices for all elements exceeding a given threshold.
- Test the function on an array where some rows have no elements greater than 10 and handle the empty case.
- Compare the indices obtained from np.where with those determined by iterating through the array manually.
Go to:
PREV : Sort Pairs by Names
NEXT : Save Array to Text File
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.