NumPy: Create a new array of 3*5, filled with 2
3x5 Array Filled with 2s
Write a NumPy program to create a new array of 3*5, filled with 2.
Pictorial Presentation:
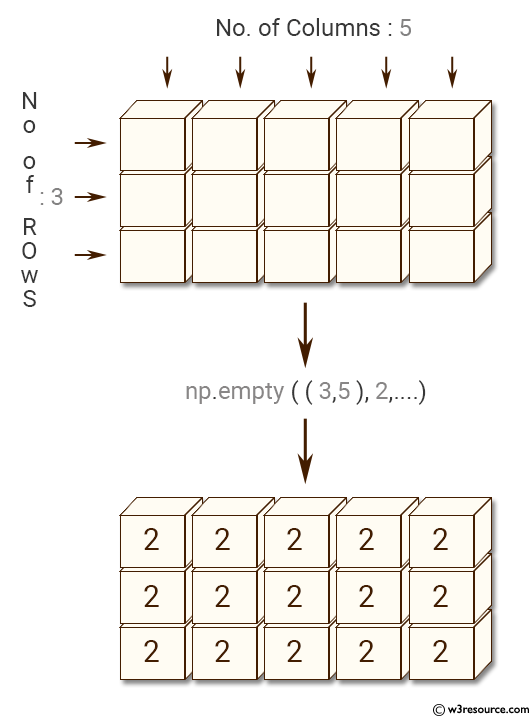
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 3x5 array filled with 2s using np.full method and specifying the data type as unsigned int
x = np.full((3, 5), 2, dtype=np.uint)
print(x)
# Creating a 3x5 array filled with 2s using np.ones method and multiplying the array with 2 to get the desired values
# Specifying the data type as unsigned int
y = np.ones([3, 5], dtype=np.uint) * 2
print(y)
Sample Output:
[[2 2 2 2 2] [2 2 2 2 2] [2 2 2 2 2]] [[2 2 2 2 2] [2 2 2 2 2] [2 2 2 2 2]]
Explanation:
In the above code -
x = np.full((3, 5), 2, dtype=np.uint): The present line creates a 3x5 NumPy array x filled with the value 2 and with data type np.uint (unsigned integer).
y = np.ones([3, 5], dtype=np.uint) *2: The present line first creates a 3x5 NumPy array filled with ones and with data type np.uint using the np.ones() function. Then, it multiplies the array by 2, effectively filling it with the value 2.
Python-Numpy Code Editor:
Previous: Write a NumPy program to change the data type of an array.Next: Write a NumPy program to create an array of 10's with the same shape and type of an given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics