NumPy: Create a 1-D array going from 0 to 50
1D Array (0–50) & (10–50)
Write a NumPy program to create a 1-D array with values from 0 to 50 and an array from 10 to 50.
Pictorial Presentation:
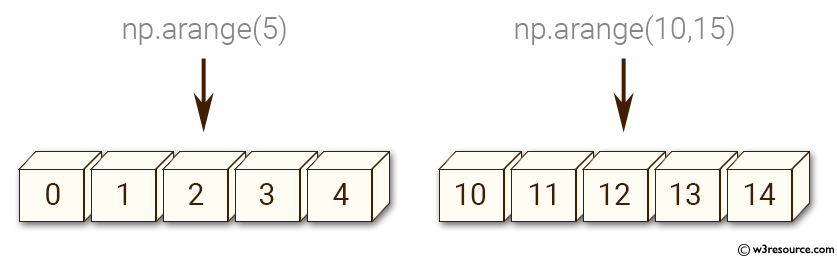
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating an array from 0 to 49 using arange
x = np.arange(50)
# Printing the array from 0 to 49
print("Array from 0 to 50:")
print(x)
# Creating an array from 10 to 49 using arange
x = np.arange(10, 50)
# Printing the array from 10 to 49
print("Array from 10 to 50:")
print(x)
Sample Output:
Array from 0 to 50: [ 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 2 3 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 4 8 49] Array from 10 to 50: [10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 3 3 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49]
Explanation:
In the above code -
x = np.arange(50): The present line creates a NumPy array x using the np.arange() function. The function takes one argument, which is the stop value. It generates a sequence of integers starting from 0 (inclusive) up to, but not including, the stop value (in this case, 50). The resulting array will contain integers from 0 to 49.
x = np.arange(10, 50): The present line creates another NumPy array x using the np.arange() function. This time, the function takes two arguments: the start value (10) and the stop value (50). It generates a sequence of integers from the start value (inclusive) up to, but not including, the stop value. The resulting array will contain integers from 10 to 49.
For more Practice: Solve these Related Problems:
- Generate two 1D arrays: one with values from 0 to 49 and another with values from 10 to 49 using np.arange.
- Create a function that returns both arrays and then computes their set difference.
- Validate the starting and ending values of both arrays to ensure correct ranges.
- Compare the two arrays element-wise to determine overlapping and non-overlapping values.
Go to:
PREV : 2D Array with Custom Diagonal Values
NEXT : 30 Evenly Spaced Values (2.5–6.5)
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.