NumPy: Move axes of an array to new positions
Move Array Axes to Alternate Positions
Write a NumPy program to move array axes to alternate positions. Other axes remain in their original order.
Pictorial Presentation:
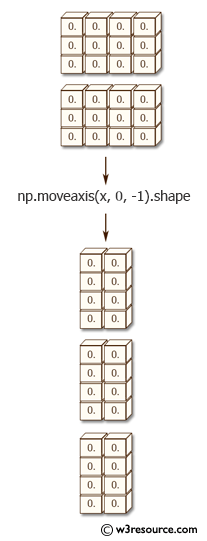
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array filled with zeros of shape (2, 3, 4)
x = np.zeros((2, 3, 4))
# Moving the axis at index 0 to the last position (-1)
# The shape of the array after moving the axis from index 0 to index -1
print(np.moveaxis(x, 0, -1).shape)
# Moving the axis at the last position (-1) to index 0
# The shape of the array after moving the axis from index -1 to index 0
print(np.moveaxis(x, -1, 0).shape)
Sample Output:
(3, 4, 2) (4, 2, 3)
Explanation:
x = np.zeros((2, 3, 4)): This line creates a 3D array x with the given shape (2, 3, 4), filled with zeros.
print(np.moveaxis(x, 0, -1).shape): The np.moveaxis() function takes three arguments: the input array, the source axis, and the destination axis. In this case, it moves axis 0 (the first axis) to the last position (-1). As a result, the shape of the new array is (3, 4, 2).
print(np.moveaxis(x, -1, 0).shape): This line moves the last axis (-1) to the first position (0). As a result, the shape of the new array is (4, 2, 3).
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics