NumPy: Insert a new axis within a 2-D array
NumPy: Array Object Exercise-56 with Solution
Write a NumPy program to insert a new axis within a 2-D array.
2-D array of shape (3, 4).
New shape will be will be (3, 1, 4).
Pictorial Presentation:
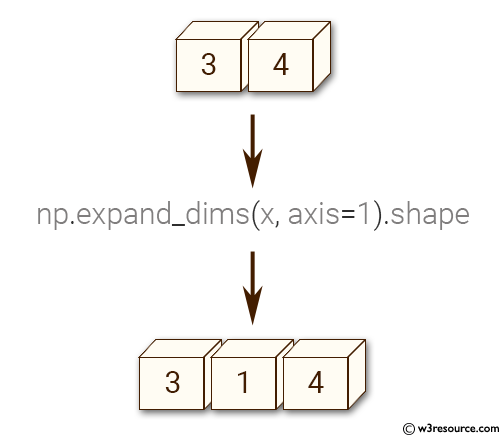
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 3x4 array of zeros
x = np.zeros((3, 4))
# Expanding the dimensions of the array x along axis 1 and storing the shape of the result in y
y = np.expand_dims(x, axis=1).shape
# Printing the shape of the expanded array
print(y)
Sample Output:
(3, 1, 4)
Explanation:
In the above code –
x = np.zeros((3, 4)): This line creates a 2D array x with shape (3, 4) filled with zeros.
y = np.expand_dims(x, axis=1).shape: The np.expand_dims() function takes the input array x and adds a new axis along the specified axis, in this case, axis=1. The original shape of x is (3, 4), and after expanding the dimensions, the new shape becomes (3, 1, 4).
print(y): Here print(y) prints the shape of the newly expanded array, which is (3, 1, 4).
Python-Numpy Code Editor:
Previous: Write a NumPy program to view inputs as arrays with at least two dimensions, three dimensions.
Next: Write a NumPy program to remove single-dimensional entries from a specified shape.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/numpy/python-numpy-exercise-56.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics