NumPy: Convert two 1-D arrays into a 2-D array
Depth-Wise Conversion of 1D to 2D
Write a NumPy program to convert (in sequence depth wise (along the third axis)) two 1-D arrays into a 2-D array.
Sample array: (10,20,30), (40,50,60)
Pictorial Presentation:
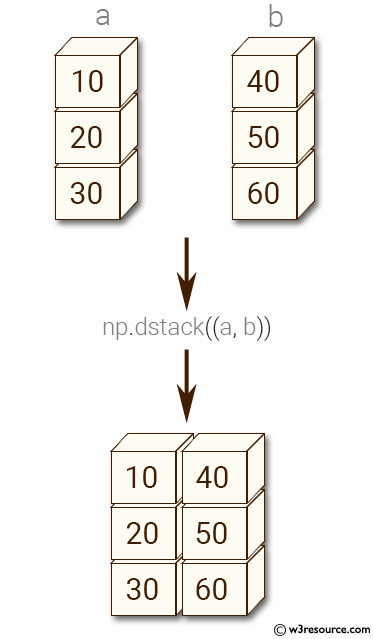
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating NumPy arrays 'a' and 'b' with vertical shapes
a = np.array([[10], [20], [30]])
b = np.array([[40], [50], [60]])
# Stacking arrays 'a' and 'b' along the third axis using np.dstack
c = np.dstack((a, b))
# Printing the resulting array 'c'
print(c)
Sample Output:
[[[10 40]] [[20 50]] [[30 60]]]
Explanation:
‘a = np.array([[10],[20],[30]])’ creates a 2D array a with shape (3, 1).
‘b = np.array([[40],[50],[60]])’ This line creates another 2D array b with shape (3, 1).
c = np.dstack((a, b)): The np.dstack() function is used to stack the two arrays ‘a’ and ‘b’ depth-wise along the third axis. The resulting array ‘c’ has the shape (3, 1, 2), where the first depth layer contains the elements of a and the second depth layer contains the elements of ‘b’.
Python-Numpy Code Editor:
Previous: Write a NumPy program to convert 1-D arrays as columns into a 2-D array.Next: Write a NumPy program to split an array of 14 elements into 3 arrays, each of which has 2, 4, and 8 elements in the original order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics