NumPy: Get the number of nonzero elements in an array
Count Non-Zero Elements in Array
Write a NumPy program to get the number of non-zero elements in an array.
Pictorial Presentation:
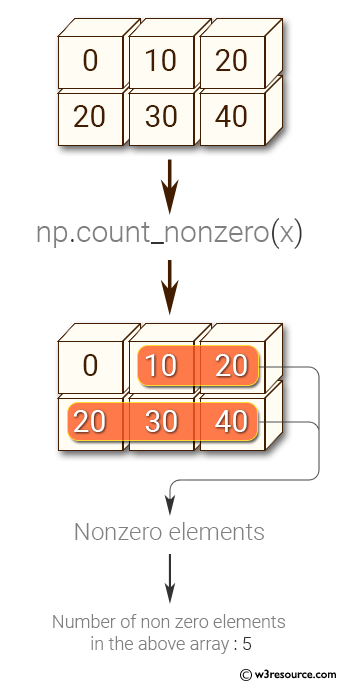
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 2x3 NumPy array 'x' containing specific values
x = np.array([[0, 10, 20], [20, 30, 40]])
# Displaying the original 2x3 array 'x'
print("Original array:")
print(x)
# Counting the number of non-zero elements in the array 'x' using np.count_nonzero
print("Number of non-zero elements in the above array:")
print(np.count_nonzero(x))
Sample Output:
Original array: [[ 0 10 20] [20 30 40]] Number of non zero elements in the above array: 5
Explanation:
In the above code –
‘x = np.array([[0, 10, 20], [20, 30, 40]])’ creates a 2D array x with the given elements.
print(np.count_nonzero(x)): The np.count_nonzero() function is used to count the number of non-zero elements in the array ‘x’. In this case, there are 5 non-zero elements in the array.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics